mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-15 11:48:23 +00:00
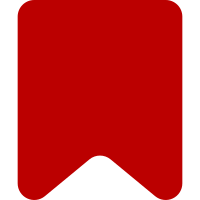
For development history of this changeset see: Id96a4b1b55e3959aab81f4ba436c5ac125f2a1bb Bug: T312528 Change-Id: I61cfdbd63f8d50b072ada05927a134686fdd53d3
51 lines
1.6 KiB
PHP
51 lines
1.6 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests\TexVC\Nodes;
|
|
|
|
use ArgumentCountError;
|
|
use MediaWiki\Extension\Math\TexVC\Nodes\ChemWord;
|
|
use MediaWiki\Extension\Math\TexVC\Nodes\Literal;
|
|
use MediaWikiUnitTestCase;
|
|
use RuntimeException;
|
|
use TypeError;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\TexVC\Nodes\ChemWord
|
|
*/
|
|
class ChemWordTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testEmptyChemWord() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new ChemWord();
|
|
throw new ArgumentCountError( 'Should not create an empty ChemWord' );
|
|
}
|
|
|
|
public function testOneArgumentChemWord() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new ChemWord( new Literal( 'a' ) );
|
|
throw new ArgumentCountError( 'Should not create a ChemWord with one argument' );
|
|
}
|
|
|
|
public function testIncorrectTypeChemWord() {
|
|
$this->expectException( TypeError::class );
|
|
new ChemWord( 'a', 'b' );
|
|
throw new RuntimeException( 'Should not create a ChemWord with incorrect type' );
|
|
}
|
|
|
|
public function testBasicFunctionChemWord() {
|
|
$chemWord = new ChemWord( new Literal( 'a' ), new Literal( 'b' ) );
|
|
$this->assertEquals( 'ab', $chemWord->render(), 'Should create a basic function' );
|
|
}
|
|
|
|
public function testGetters() {
|
|
$chemWord = new ChemWord( new Literal( 'a' ), new Literal( 'b' ) );
|
|
$this->assertNotEmpty( $chemWord->getLeft() );
|
|
$this->assertNotEmpty( $chemWord->getRight() );
|
|
}
|
|
|
|
public function testExtractIdentifiersBox() {
|
|
$chemWord = new ChemWord( new Literal( 'a' ), new Literal( 'b' ) );
|
|
$this->assertEquals( [], $chemWord->extractIdentifiers(), 'Should extract identifiers' );
|
|
}
|
|
}
|