mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-15 11:48:23 +00:00
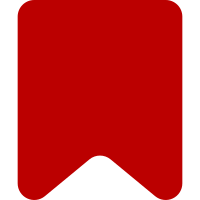
For development history of this changeset see: Id96a4b1b55e3959aab81f4ba436c5ac125f2a1bb Bug: T312528 Change-Id: I61cfdbd63f8d50b072ada05927a134686fdd53d3
51 lines
1.6 KiB
PHP
51 lines
1.6 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests\TexVC\Nodes;
|
|
|
|
use ArgumentCountError;
|
|
use MediaWiki\Extension\Math\TexVC\Nodes\ChemFun2u;
|
|
use MediaWiki\Extension\Math\TexVC\Nodes\Literal;
|
|
use MediaWikiUnitTestCase;
|
|
use TypeError;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\TexVC\Nodes\ChemFun2u
|
|
*/
|
|
class ChemFun2uTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testEmptyChemFun2u() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new ChemFun2u();
|
|
throw new ArgumentCountError( 'Should not create an empty ChemFun2u' );
|
|
}
|
|
|
|
public function testOneArgumentChemFun2u() {
|
|
$this->expectException( ArgumentCountError::class );
|
|
new ChemFun2u( 'a' );
|
|
throw new ArgumentCountError( 'Should not create a ChemFun2u with one argument' );
|
|
}
|
|
|
|
public function testIncorrectTypeChemFun2u() {
|
|
$this->expectException( TypeError::class );
|
|
new ChemFun2u( 'a', 'b', 'c' );
|
|
throw new TypeError( 'Should not create a ChemFun2u with incorrect type' );
|
|
}
|
|
|
|
public function testBasicChemFun2u() {
|
|
$fun2u = new ChemFun2u( 'a', new Literal( 'b' ), new Literal( 'c' ) );
|
|
$this->assertEquals( 'a{b}_{c}', $fun2u->render(), 'Should create a basic ChemFun2u' );
|
|
}
|
|
|
|
public function testGetters() {
|
|
$fun2u = new ChemFun2u( 'a', new Literal( 'b' ), new Literal( 'c' ) );
|
|
$this->assertNotEmpty( $fun2u->getFname() );
|
|
$this->assertNotEmpty( $fun2u->getLeft() );
|
|
$this->assertNotEmpty( $fun2u->getRight() );
|
|
}
|
|
|
|
public function testExtractIdentifiers() {
|
|
$fun2u = new ChemFun2u( 'a', new Literal( 'b' ), new Literal( 'c' ) );
|
|
$this->assertEquals( [], $fun2u->extractIdentifiers(), 'Should extract identifiers' );
|
|
}
|
|
}
|