mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-15 19:50:16 +00:00
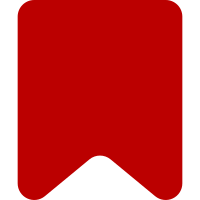
Avoid that user input is passed to the HTML output directly by using HTML methods for tag generation. This also enables additional phan checks. Change-Id: Iff584ac829c190e413a36331c53d6835a86bc0d5
62 lines
1.3 KiB
PHP
62 lines
1.3 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Math\Tests\TexVC\MMLnodes;
|
|
|
|
use MediaWiki\Extension\Math\TexVC\MMLmappings\TexConstants\Tag;
|
|
use MediaWiki\Extension\Math\TexVC\MMLnodes\MMLbase;
|
|
use MediaWikiUnitTestCase;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\TexVC\MMLnodes\MMLbase
|
|
*/
|
|
class BaseTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testEmpty() {
|
|
$this->assertEquals(
|
|
'<test/>',
|
|
( new MMLbase( 'test' ) )->getEmpty()
|
|
);
|
|
}
|
|
|
|
public function testEnd() {
|
|
$this->assertEquals(
|
|
'</test>',
|
|
( new MMLbase( 'test' ) )->getEnd()
|
|
);
|
|
}
|
|
|
|
public function testEncapsulate() {
|
|
$this->assertEquals(
|
|
'<test><script>alert(document.cookies);</script></test>',
|
|
( new MMLbase( 'test' ) )
|
|
->encapsulate( '<script>alert(document.cookies);</script>' )
|
|
);
|
|
}
|
|
|
|
public function testEncapsulateRaw() {
|
|
$this->assertEquals(
|
|
'<test><script>alert(document.cookies);</script></test>',
|
|
( new MMLbase( 'test' ) )
|
|
->encapsulateRaw( '<script>alert(document.cookies);</script>' )
|
|
);
|
|
}
|
|
|
|
public function testAttributes() {
|
|
$this->assertEquals(
|
|
'<test data-mjx-texclass="texClass1" a="b">',
|
|
( new MMLbase(
|
|
'test',
|
|
'texClass1',
|
|
[ TAG::CLASSTAG => 'texClass2', 'a' => 'b' ] ) )
|
|
->getStart()
|
|
);
|
|
}
|
|
|
|
public function testName() {
|
|
$this->assertEquals(
|
|
'test',
|
|
( new MMLbase( 'test' ) )->name()
|
|
);
|
|
}
|
|
}
|