mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-28 17:30:40 +00:00
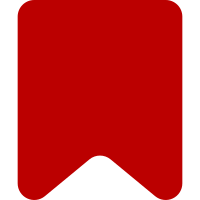
Using assertContains() with string haystacks is deprecated and will not be supported in PHPUnit 9. Refactor your test to use assertStringContainsString() or assertStringContainsStringIgnoringCase() instead. Change-Id: Ic35f3c60a7f49dfe244b87192d7f161c117b37e1
75 lines
2.2 KiB
PHP
75 lines
2.2 KiB
PHP
<?php
|
|
|
|
/**
|
|
* @covers \MathMathMLCli
|
|
*
|
|
* @group Math
|
|
*
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class MathoidCliTest extends MediaWikiTestCase {
|
|
private $goodInput = '\sin\left(\frac12x\right)';
|
|
private $badInput = '\newcommand{\text{do evil things}}';
|
|
protected static $hasMathoidCli;
|
|
|
|
public static function setUpBeforeClass() : void {
|
|
global $wgMathoidCli;
|
|
if ( is_array( $wgMathoidCli ) && is_executable( $wgMathoidCli[0] ) ) {
|
|
self::$hasMathoidCli = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Sets up the fixture, for example, opens a network connection.
|
|
* This method is called before a test is executed.
|
|
*/
|
|
protected function setUp() : void {
|
|
parent::setUp();
|
|
if ( !self::$hasMathoidCli ) {
|
|
$this->markTestSkipped( "No mathoid cli configured on server" );
|
|
}
|
|
}
|
|
|
|
public function testGood() {
|
|
$mml = new MathMathMLCli( $this->goodInput );
|
|
$input = [ 'good' => [ $mml ] ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertTrue( $mml->render(), 'assert that renders' );
|
|
$this->assertStringContainsString( '</mo>', $mml->getMathml() );
|
|
}
|
|
|
|
public function testUndefinedFunctionError() {
|
|
$mml = new MathMathMLCli( $this->badInput );
|
|
$input = [ 'bad' => [ $mml ] ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'newcommand', $mml->getLastError() );
|
|
}
|
|
|
|
public function testSyntaxError() {
|
|
$mml = new MathMathMLCli( '^' );
|
|
$input = [ 'bad' => [ $mml ] ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'SyntaxError', $mml->getLastError() );
|
|
}
|
|
|
|
public function testCeError() {
|
|
$mml = new MathMathMLCli( '\ce{H2O}' );
|
|
$input = [ 'bad' => [ $mml ] ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'SyntaxError', $mml->getLastError() );
|
|
}
|
|
|
|
public function testEmpty() {
|
|
$mml = new MathMathMLCli( '' );
|
|
$input = [ 'bad' => [ $mml ] ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that renders' );
|
|
$this->assertFalse( $mml->isTexSecure() );
|
|
$this->assertStringContainsString( 'empty', $mml->getLastError() );
|
|
}
|
|
|
|
}
|