mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-24 23:46:39 +00:00
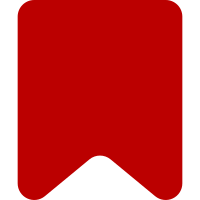
This fixes some warnings in my PHPStorm, gets rid of duplicate code, inlines constants that do not make the code easier to read, and more. Change-Id: I02f446491509043f5d3e51e26e932f76c9ecb6cf
86 lines
2.2 KiB
PHP
86 lines
2.2 KiB
PHP
<?php
|
|
/**
|
|
* MediaWiki math extension
|
|
*
|
|
* (c) 2002-2015 Tomasz Wegrzanowski, Brion Vibber, Moritz Schubotz,
|
|
* and other MediaWiki contributors
|
|
* GPLv2 license; info in main package.
|
|
*
|
|
* @author Moritz Schubotz
|
|
*/
|
|
|
|
class MathInputCheckRestbase extends MathInputCheck {
|
|
private $restbaseInterface;
|
|
|
|
/**
|
|
* Default constructor
|
|
* (performs no checking)
|
|
* @param string $tex the TeX input string to be checked
|
|
* @param bool $displayStyle
|
|
*/
|
|
public function __construct( $tex = '', $displayStyle = true ) {
|
|
parent::__construct( $tex );
|
|
$this->restbaseInterface = new MathRestbaseInterface( $tex, $displayStyle );
|
|
}
|
|
|
|
/**
|
|
* @see https://phabricator.wikimedia.org/T119300
|
|
* @param stdClass $e
|
|
* @param MathRenderer $errorRenderer
|
|
* @return string
|
|
*/
|
|
public function errorObjectToHtml( stdClass $e, $errorRenderer = null ) {
|
|
if ( $errorRenderer === null ) {
|
|
$errorRenderer = new MathSource( $this->inputTeX );
|
|
}
|
|
if ( isset( $e->error->message ) ){
|
|
if ( $e->error->message === 'Illegal TeX function' ) {
|
|
return $errorRenderer->getError( 'math_unknown_function', $e->error->found );
|
|
} elseif ( preg_match( '/Math extension/', $e->error->message ) ) {
|
|
$names = MathHooks::getMathNames();
|
|
$mode = $names['mathml'];
|
|
try {
|
|
$host = $this->restbaseInterface->getUrl( '' );
|
|
}
|
|
catch ( Exception $ignore ) {
|
|
$host = 'invalid';
|
|
}
|
|
$msg = $e->error->message;
|
|
return $errorRenderer->getError( 'math_invalidresponse', $mode, $host, $msg );
|
|
}
|
|
return $errorRenderer->getError( 'math_syntax_error' );
|
|
}
|
|
return $errorRenderer->getError( 'math_unknown_error' );
|
|
}
|
|
/**
|
|
* @return boolean
|
|
*/
|
|
public function isValid() {
|
|
return $this->restbaseInterface->checkTeX();
|
|
}
|
|
|
|
/**
|
|
* Some TeX checking programs may return
|
|
* a modified tex string after having checked it.
|
|
* You can get the altered tex string with this method
|
|
* @return string A valid Tex string
|
|
*/
|
|
public function getValidTex() {
|
|
return $this->restbaseInterface->getCheckedTex();
|
|
}
|
|
|
|
|
|
/**
|
|
* Returns the string of the last error.
|
|
* @return string
|
|
*/
|
|
public function getError() {
|
|
$err = $this->restbaseInterface->getError();
|
|
if ( $err === null ){
|
|
return null;
|
|
}
|
|
return $this->errorObjectToHtml( $err );
|
|
}
|
|
|
|
}
|