mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Math
synced 2024-11-13 17:56:59 +00:00
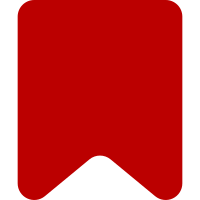
Add doc-typehints to class properties found by the PropertyDocumentation sniff to improve the documentation and to remove the exclusion of the sniff. The activated sniff avoids that new code is missing doc-typehints or real-typehints. Change-Id: Id7fcfd086cdbb3f040091e1d1a81472e7a524091
80 lines
2.3 KiB
PHP
80 lines
2.3 KiB
PHP
<?php
|
|
|
|
use MediaWiki\Extension\Math\MathMathMLCli;
|
|
|
|
/**
|
|
* @covers \MediaWiki\Extension\Math\MathMathMLCli
|
|
*
|
|
* @group Math
|
|
*
|
|
* @license GPL-2.0-or-later
|
|
*/
|
|
class MathoidCliTest extends MediaWikiIntegrationTestCase {
|
|
/** @var string */
|
|
private $goodInput = '\sin\left(\frac12x\right)';
|
|
/** @var string */
|
|
private $badInput = '\newcommand{\text{do evil things}}';
|
|
/** @var true|null */
|
|
protected static $hasMathoidCli;
|
|
|
|
public static function setUpBeforeClass(): void {
|
|
global $wgMathoidCli;
|
|
if ( is_array( $wgMathoidCli ) && is_executable( $wgMathoidCli[0] ) ) {
|
|
self::$hasMathoidCli = true;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Sets up the fixture, for example, opens a network connection.
|
|
* This method is called before a test is executed.
|
|
*/
|
|
protected function setUp(): void {
|
|
parent::setUp();
|
|
if ( !self::$hasMathoidCli ) {
|
|
$this->markTestSkipped( "No mathoid cli configured on server" );
|
|
}
|
|
}
|
|
|
|
public function testGood() {
|
|
$mml = new MathMathMLCli( $this->goodInput );
|
|
$input = [ 'good' => $mml ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertTrue( $mml->render(), 'assert that renders' );
|
|
$this->assertStringContainsString( '</mo>', $mml->getMathml() );
|
|
}
|
|
|
|
public function testUndefinedFunctionError() {
|
|
$mml = new MathMathMLCli( $this->badInput );
|
|
$input = [ 'bad' => $mml ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'newcommand', $mml->getLastError() );
|
|
}
|
|
|
|
public function testSyntaxError() {
|
|
$mml = new MathMathMLCli( '^' );
|
|
$input = [ 'bad' => $mml ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'SyntaxError', $mml->getLastError() );
|
|
}
|
|
|
|
public function testCeError() {
|
|
$mml = new MathMathMLCli( '\ce{H2O}' );
|
|
$input = [ 'bad' => $mml ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that fails' );
|
|
$this->assertStringContainsString( 'SyntaxError', $mml->getLastError() );
|
|
}
|
|
|
|
public function testEmpty() {
|
|
$mml = new MathMathMLCli( '' );
|
|
$input = [ 'bad' => $mml ];
|
|
MathMathMLCli::batchEvaluate( $input );
|
|
$this->assertFalse( $mml->render(), 'assert that renders' );
|
|
$this->assertFalse( $mml->isTexSecure() );
|
|
$this->assertStringContainsString( 'empty', $mml->getLastError() );
|
|
}
|
|
|
|
}
|