mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/LoginNotify
synced 2024-11-13 17:53:12 +00:00
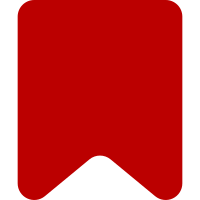
* Remove notification attributes such as title-message, unused since the initial commit since it used the new (2015) formatter system. * isKnownSystemSlow() is always called with a third parameter, and it doesn't seem to be nullable in callers. * Yes, most of the things make sense. * Add reason why CheckUser has no cuc_ip_hex index. * Use foreach * Too late to truncate the hash now Change-Id: I310bc53ba881842845b9358309954f89c355f81c
92 lines
2.3 KiB
PHP
92 lines
2.3 KiB
PHP
<?php
|
|
|
|
namespace LoginNotify;
|
|
|
|
use MediaWiki\Extension\Notifications\AttributeManager;
|
|
use MediaWiki\Extension\Notifications\Hooks\BeforeCreateEchoEventHook;
|
|
use MediaWiki\Extension\Notifications\Hooks\EchoGetBundleRulesHook;
|
|
use MediaWiki\Extension\Notifications\Model\Event;
|
|
use MediaWiki\Extension\Notifications\UserLocator;
|
|
|
|
/**
|
|
* Hooks from Echo extension,
|
|
* which is optional to use with this extension.
|
|
*/
|
|
class EchoHooks implements
|
|
BeforeCreateEchoEventHook,
|
|
EchoGetBundleRulesHook
|
|
{
|
|
/**
|
|
* Add LoginNotify events to Echo
|
|
*
|
|
* @param string[] &$notifications Array of Echo notifications
|
|
* @param string[] &$notificationCategories Array of Echo notification categories
|
|
* @param string[] &$icons Array of icon details
|
|
*/
|
|
public function onBeforeCreateEchoEvent(
|
|
array &$notifications,
|
|
array &$notificationCategories,
|
|
array &$icons
|
|
) {
|
|
global $wgLoginNotifyEnableOnSuccess, $wgNotifyTypeAvailabilityByCategory;
|
|
|
|
$icons['LoginNotify-user-avatar'] = [
|
|
'path' => 'LoginNotify/UserAvatar.svg'
|
|
];
|
|
|
|
$notificationCategories['login-fail'] = [
|
|
'priority' => 7,
|
|
'tooltip' => 'echo-pref-tooltip-login-fail',
|
|
];
|
|
|
|
$loginBase = [
|
|
AttributeManager::ATTR_LOCATORS => [
|
|
[ [ UserLocator::class, 'locateEventAgent' ] ],
|
|
],
|
|
'canNotifyAgent' => true,
|
|
'category' => 'login-fail',
|
|
'group' => 'negative',
|
|
'presentation-model' => PresentationModel::class,
|
|
'icon' => 'LoginNotify-user-avatar',
|
|
'immediate' => true,
|
|
];
|
|
$notifications['login-fail-new'] = [
|
|
'bundle' => [
|
|
'web' => true,
|
|
'expandable' => false
|
|
]
|
|
] + $loginBase;
|
|
$notifications['login-fail-known'] = [
|
|
'bundle' => [
|
|
'web' => true,
|
|
'expandable' => false
|
|
]
|
|
] + $loginBase;
|
|
if ( $wgLoginNotifyEnableOnSuccess ) {
|
|
$notificationCategories['login-success'] = [
|
|
'priority' => 7,
|
|
'tooltip' => 'echo-pref-tooltip-login-success',
|
|
];
|
|
$notifications['login-success'] = [
|
|
'category' => 'login-success',
|
|
] + $loginBase;
|
|
$wgNotifyTypeAvailabilityByCategory['login-success'] = [
|
|
'web' => false,
|
|
'email' => true,
|
|
];
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @param Event $event
|
|
* @param string &$bundleString
|
|
*/
|
|
public function onEchoGetBundleRules( Event $event, string &$bundleString ) {
|
|
switch ( $event->getType() ) {
|
|
case 'login-fail-new':
|
|
$bundleString = 'login-fail';
|
|
break;
|
|
}
|
|
}
|
|
}
|