mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Linter
synced 2024-12-01 02:46:24 +00:00
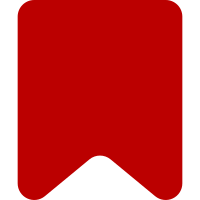
Currently we select 20 rows, and return the accurate count if it's less than that, so up to 19 rows. Since we want to return an accurate count if it's 20 rows or less, select one more row, 21, so we can differentiate between only having 20 result rows or hitting the limit. This is the same technique used in MediaWiki's Pager system. Change-Id: I50fa96238eb4c7178414ee92c53799fd69520926
138 lines
5.2 KiB
PHP
138 lines
5.2 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
namespace MediaWiki\Linter\Test;
|
|
|
|
use MediaWiki\Linter\Database;
|
|
use MediaWiki\Linter\LintError;
|
|
use MediaWikiTestCase;
|
|
|
|
/**
|
|
* @group Database
|
|
* @covers MediaWiki\Linter\Database
|
|
*/
|
|
class DatabaseTest extends MediaWikiTestCase {
|
|
public function testConstructor() {
|
|
$this->assertInstanceOf( Database::class, new Database( 5 ) );
|
|
}
|
|
|
|
private function getDummyLintErrors() {
|
|
return [
|
|
new LintError(
|
|
'fostered', [ 0, 10 ], []
|
|
),
|
|
new LintError(
|
|
'obsolete-tag', [ 15, 20 ], [ 'name' => 'big' ]
|
|
),
|
|
];
|
|
}
|
|
|
|
private function assertSetForPageResult( $result, $deleted, $added ) {
|
|
$this->assertArrayHasKey( 'deleted', $result );
|
|
$this->assertEquals( $deleted, $result['deleted'] );
|
|
$this->assertArrayHasKey( 'added', $result );
|
|
$this->assertEquals( $added, $result['added'] );
|
|
}
|
|
|
|
private function assertLintErrorsEqual( $expected, $actual ) {
|
|
$expectedIds = array_map( static function ( LintError $error ) {
|
|
return $error->id();
|
|
}, $expected );
|
|
$actualIds = array_map( static function ( LintError $error ) {
|
|
return $error->id();
|
|
}, $actual );
|
|
$this->assertArrayEquals( $expectedIds, $actualIds );
|
|
}
|
|
|
|
private function createManyLintErrors( $lintDb, $errorCount ) {
|
|
$manyLintErrors = [];
|
|
for ( $i = 0; $i < $errorCount; $i++ ) {
|
|
$manyLintErrors[] = new LintError(
|
|
'obsolete-tag', [ 15, 20 + $i ], [ 'name' => 'big' ]
|
|
);
|
|
}
|
|
$lintDb->setForPage( $manyLintErrors );
|
|
}
|
|
|
|
public function testSetForPage() {
|
|
$lintDb = new Database( 5 );
|
|
$dummyErrors = $this->getDummyLintErrors();
|
|
$result = $lintDb->setForPage( $dummyErrors );
|
|
$this->assertSetForPageResult( $result, [], [ 'fostered' => 1, 'obsolete-tag' => 1 ] );
|
|
$this->assertLintErrorsEqual( $dummyErrors, $lintDb->getForPage() );
|
|
|
|
// Accurate low error count values should match for both methods
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertEquals( $resultTotals, $resultEstimatedTotals );
|
|
|
|
// Should delete the second error
|
|
$result2 = $lintDb->setForPage( [ $dummyErrors[0] ] );
|
|
$this->assertSetForPageResult( $result2, [ 'obsolete-tag' => 1 ], [] );
|
|
$this->assertLintErrorsEqual( [ $dummyErrors[0] ], $lintDb->getForPage() );
|
|
|
|
// Accurate low error count values should match for both methods
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertEquals( $resultTotals, $resultEstimatedTotals );
|
|
|
|
// Insert the second error, delete the first
|
|
$result3 = $lintDb->setForPage( [ $dummyErrors[1] ] );
|
|
$this->assertSetForPageResult( $result3, [ 'fostered' => 1 ], [ 'obsolete-tag' => 1 ] );
|
|
$this->assertLintErrorsEqual( [ $dummyErrors[1] ], $lintDb->getForPage() );
|
|
|
|
// Delete the second (only) error
|
|
$result4 = $lintDb->setForPage( [] );
|
|
$this->assertSetForPageResult( $result4, [ 'obsolete-tag' => 1 ], [] );
|
|
$this->assertLintErrorsEqual( [], $lintDb->getForPage() );
|
|
|
|
// Accurate low error count values should match for both methods
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertEquals( $resultTotals, $resultEstimatedTotals );
|
|
|
|
// For error counts <= MAX_ACCURATE_COUNT, both error
|
|
// count methods should return the same count.
|
|
self::createManyLintErrors( $lintDb, Database::MAX_ACCURATE_COUNT );
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertEquals( $resultTotals, $resultEstimatedTotals );
|
|
|
|
// For error counts greater than MAX_ACCURATE_COUNT, both error
|
|
// count methods should NOT return the same count in this test scenario
|
|
// because previously added and deleted records will be included
|
|
// in the estimated count which is normal.
|
|
self::createManyLintErrors( $lintDb, Database::MAX_ACCURATE_COUNT + 1 );
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertNotEquals( $resultTotals, $resultEstimatedTotals );
|
|
|
|
// For error counts greatly above the MAX_ACCURATE_COUNT, the estimated
|
|
// count method should return a greater count in this test scenario
|
|
// because previously added and deleted records will be included
|
|
// in the estimated count which is normal.
|
|
self::createManyLintErrors( $lintDb, Database::MAX_ACCURATE_COUNT * 10 );
|
|
$resultTotals = $lintDb->getTotalsForPage();
|
|
$resultEstimatedTotals = $lintDb->getTotals();
|
|
$this->assertGreaterThan( $resultTotals, $resultEstimatedTotals );
|
|
}
|
|
|
|
}
|