mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Gadgets
synced 2024-11-12 01:01:48 +00:00
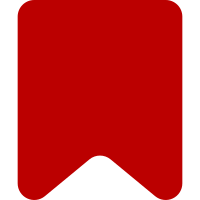
For each item, either display human-readable and translated text, or display a technical non-translatable identifier as `<code>`, with optional localised text in the title attribute. * Re-format "rights" as a sentence instead of a bullet list. It was the only one using a bullet list, which made it feel a bit long. * Re-format "actions" as `<code>` since they are not localised. * Re-format "contentModels" as `<code>`, and add the localised display name in a title attribute, same as we do with "rights" already. ContentHandler::getLocalizedName() is also used already on Special:ChangeContentModel. * Fix "contentModels" to set `needLineBreakAfter = true`, otherwise if a gadget also sets "supportsUrlLoad", then that sentence is appended to the previous line. Update phrasing and sorting to be consistent everywhere, and adopt native PHP types where possible. In most cases, I made things alphabetical, with the exception of Special:Gadgets user interface output, and Gadget class methods, which both follow the order of most recently added feature last (rights, skins, actions, namespaces, contentmodels, categories). Highlights: * Fix namespace IDs type. These can be strings when they are parsed from the gadget definition text, not always integers. * Add explicit default for 'category'. In theory not needed because MediaWikiGadgetsDefinitionRepo has a `$section = '';` default, and MediaWikiGadgetsJsonRepo uses GadgetDefinitionContentHandler where `category: ''` is part of both the initial page content, as well as merged via getDefaultMetadata. This default benefits simpler test cases, and static analysis, since the Gadget class constructor does not (yet) require it. Without this, getCategory() could TypeError due to returning null. Bug: T63007 Change-Id: I3b2c72a6424d520431d03761d23a5a222518ce3d
71 lines
2.1 KiB
PHP
71 lines
2.1 KiB
PHP
<?php
|
|
/**
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\Gadgets;
|
|
|
|
use MediaWiki\Output\OutputPage;
|
|
use MediaWiki\User\User;
|
|
use Skin;
|
|
|
|
/**
|
|
* @author Siddharth VP
|
|
*/
|
|
class GadgetLoadConditions {
|
|
/** @var User */
|
|
private $user;
|
|
/** @var Skin */
|
|
private $skin;
|
|
/** @var string */
|
|
private $action;
|
|
/** @var int */
|
|
private $namespace;
|
|
/** @var string[] */
|
|
private $categories;
|
|
/** @var string */
|
|
private $contentModel;
|
|
/** @var string|null */
|
|
private $withGadgetParam;
|
|
|
|
/**
|
|
* @param OutputPage $out
|
|
*/
|
|
public function __construct( OutputPage $out ) {
|
|
$this->user = $out->getUser();
|
|
$this->skin = $out->getSkin();
|
|
$this->action = $out->getContext()->getActionName();
|
|
$this->namespace = $out->getTitle()->getNamespace();
|
|
$this->categories = $out->getCategories();
|
|
$this->contentModel = $out->getTitle()->getContentModel();
|
|
$this->withGadgetParam = $out->getRequest()->getRawVal( 'withgadget' );
|
|
}
|
|
|
|
public function check( Gadget $gadget ): bool {
|
|
$urlLoad = $this->withGadgetParam === $gadget->getName() && $gadget->supportsUrlLoad();
|
|
|
|
return ( $gadget->isEnabled( $this->user ) || $urlLoad )
|
|
&& $gadget->isAllowed( $this->user )
|
|
&& $gadget->isActionSupported( $this->action )
|
|
&& $gadget->isSkinSupported( $this->skin )
|
|
&& $gadget->isNamespaceSupported( $this->namespace )
|
|
&& $gadget->isCategorySupported( $this->categories )
|
|
&& $gadget->isContentModelSupported( $this->contentModel );
|
|
}
|
|
}
|