mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Gadgets
synced 2024-11-12 01:01:48 +00:00
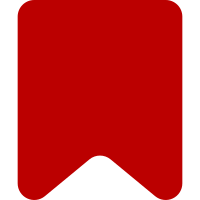
GadgetRepo is an abstract class based off of the implementation in the RL2 branch. It is a singleton that provides basic methods to construct and interact with Gadget objects. The MediaWikiGadgetsDefinition class is an implementation of GadgetsRepo that parses the "MediaWiki:Gadgets-definition" page for gadget definitions. Tests were left in place to demonstrate that no functional changes have been made aside from relocation of code. Some tests should be moved to separate files in the future. Bug: T106176 Change-Id: I3e802889f6f495783f4dbac65c2a8cefa824a778
64 lines
1.3 KiB
PHP
64 lines
1.3 KiB
PHP
<?php
|
|
|
|
abstract class GadgetRepo {
|
|
|
|
/**
|
|
* @var GadgetRepo|null
|
|
*/
|
|
private static $instance;
|
|
|
|
/**
|
|
* Get the ids of the gadgets provided by this repository
|
|
*
|
|
* @return string[]
|
|
*/
|
|
abstract public function getGadgetIds();
|
|
|
|
/**
|
|
* Get the Gadget object for a given gadget id
|
|
*
|
|
* @param string $id
|
|
* @throws InvalidArgumentException
|
|
* @return Gadget
|
|
*/
|
|
abstract public function getGadget( $id );
|
|
|
|
/**
|
|
* Get a list of gadgets sorted by category
|
|
*
|
|
* @return array array( 'category' => array( 'name' => $gadget ) )
|
|
*/
|
|
public function getStructuredList() {
|
|
$list = array();
|
|
foreach ( $this->getGadgetIds() as $id ) {
|
|
$gadget = $this->getGadget( $id );
|
|
$list[$gadget->getCategory()][$gadget->getName()] = $gadget;
|
|
}
|
|
|
|
return $list;
|
|
}
|
|
|
|
/**
|
|
* Get the configured default GadgetRepo. Currently
|
|
* this hardcodes MediaWikiGadgetsDefinitionRepo since
|
|
* that is the only implementation
|
|
*
|
|
* @return GadgetRepo
|
|
*/
|
|
public static function singleton() {
|
|
if ( self::$instance === null ) {
|
|
self::$instance = new MediaWikiGadgetsDefinitionRepo();
|
|
}
|
|
return self::$instance;
|
|
}
|
|
|
|
/**
|
|
* Should only be used by unit tests
|
|
*
|
|
* @param GadgetRepo|null $repo
|
|
*/
|
|
public static function setSingleton( $repo = null ) {
|
|
self::$instance = $repo;
|
|
}
|
|
}
|