mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Gadgets
synced 2024-11-24 15:33:42 +00:00
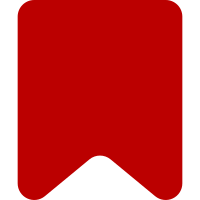
== Motivation == On a local dev wiki and in CI, where no gadgets are defined yet, fetchStructuredList() is called on every load.php request and doing uncached database look ups. This is because T39228 (stale cache after Gadgets-definition was empty or created) was fixed by disabling the cache until the page exists. This seems like a poor solution, and commit I3092bcb162d032 recognises that the problem was not understood at the time. I propose to instead cache it always and purge it when the page is modified in any way. Cold calling fetchStructuredList() accounted for ~170ms of ~400ms when trying out debug v2 (T85805), thus making it significantly slower and causing a chain of dozens of requests to pile up. == Previously == * Legacy repo (MediaWikiGadgetsDefinitionRepo) only implemented handlePageUpdate. * handlePageUpdate was called from onPageSaveComplete for both any page edit, and for creations in the experimental namespace. * The experimental GadgetDefinitionNamespaceRepo is based on ContentHandler rather than global hooks. This system does not have a create-specific callback. It called update for edit/create, and delete for delete. The experimental repo relied on create being called from the global hook for the legacy repo, and update was then called twice. * There was no global hook for onPageDeleteComplete, thus the legacy repo did not get purges. == Changes == * Add onPageDeleteComplete hook to fix purges after deletion, with the legacy repo now implementing handlePageDeletion() and doing the same as handlePageUpdate(). * Fix handlePageUpdate() docs to reflect that it covers page create, since onPageSaveComplete() called it either way. * Fix experimental repo to include its namespace purge in its handlePageUpdate() method. * Get rid of now-redundant handlePageCreation(). * Get rid of handlePageDeletion() since its implementations would now be identical to handlePageUpdate(). All these hooks and handle*() methods are just for a memc key holding gadget metadata. We don't need to microoptimise this on a per-page basis. Gadget edits are rare enough that purging them as a whole each time is fine. We do the same in MediaWiki core with ResourceLoaderGadgetsModule already. Bug: T85805 Change-Id: Ib27fd34fbfe7a75c851602c8a93a2e3e1f2c38a0
48 lines
1.3 KiB
PHP
48 lines
1.3 KiB
PHP
<?php
|
|
/**
|
|
* Copyright 2014
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License along
|
|
* with this program; if not, write to the Free Software Foundation, Inc.,
|
|
* 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301, USA.
|
|
* http://www.gnu.org/copyleft/gpl.html
|
|
*
|
|
* @file
|
|
*/
|
|
|
|
namespace MediaWiki\Extension\Gadgets\Content;
|
|
|
|
use DataUpdate;
|
|
use MediaWiki\Extension\Gadgets\GadgetRepo;
|
|
use MediaWiki\Linker\LinkTarget;
|
|
|
|
/**
|
|
* DataUpdate to run whenever a page in the Gadget definition
|
|
* is deleted.
|
|
*/
|
|
class GadgetDefinitionDeletionUpdate extends DataUpdate {
|
|
/**
|
|
* Page that was deleted
|
|
* @var LinkTarget
|
|
*/
|
|
private $target;
|
|
|
|
public function __construct( LinkTarget $target ) {
|
|
$this->target = $target;
|
|
}
|
|
|
|
public function doUpdate() {
|
|
GadgetRepo::singleton()->handlePageUpdate( $this->target );
|
|
}
|
|
}
|