mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Gadgets
synced 2024-11-15 03:23:51 +00:00
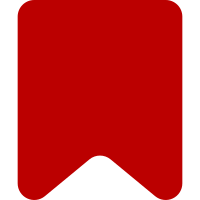
Removing the links to edit the UI messages means it's necessary to dig around and guess at names to update the gadget descriptions. IMHO this is pretty terrible, and definitely a step backwards in usability.
104 lines
2.5 KiB
PHP
104 lines
2.5 KiB
PHP
<?php
|
|
/**
|
|
* Special:Gadgets, provides a preview of MediaWiki:Gadgets.
|
|
*
|
|
* @addtogroup SpecialPage
|
|
* @author Daniel Kinzler, brightbyte.de
|
|
* @copyright © 2007 Daniel Kinzler
|
|
* @license GNU General Public License 2.0 or later
|
|
*/
|
|
|
|
if( !defined( 'MEDIAWIKI' ) ) {
|
|
echo( "not a valid entry point.\n" );
|
|
die( 1 );
|
|
}
|
|
|
|
/**
|
|
*
|
|
*/
|
|
class SpecialGadgets extends SpecialPage {
|
|
|
|
/**
|
|
* Constructor
|
|
*/
|
|
function __construct() {
|
|
SpecialPage::SpecialPage( 'Gadgets', '', true );
|
|
}
|
|
|
|
/**
|
|
* Main execution function
|
|
* @param $par Parameters passed to the page
|
|
*/
|
|
function execute( $par ) {
|
|
global $wgOut, $wgUser, $wgLang, $wgContLang;
|
|
|
|
wfLoadExtensionMessages( 'Gadgets' );
|
|
$skin = $wgUser->getSkin();
|
|
|
|
$this->setHeaders();
|
|
$wgOut->setPagetitle( wfMsg( "gadgets-title" ) );
|
|
$wgOut->addWikiText( wfMsg( "gadgets-pagetext" ) );
|
|
|
|
$gadgets = wfLoadGadgetsStructured();
|
|
if ( !$gadgets ) return;
|
|
|
|
$lang = "";
|
|
if ( $wgLang->getCode() != $wgContLang->getCode() ) {
|
|
$lang = "/" . $wgLang->getCode();
|
|
}
|
|
|
|
$listOpen = false;
|
|
|
|
$msgOpt = array( 'parseinline', 'parsemag' );
|
|
|
|
foreach ( $gadgets as $section => $entries ) {
|
|
if ( $section !== false && $section !== '' ) {
|
|
$t = Title::makeTitleSafe( NS_MEDIAWIKI, "Gadget-section-$section$lang" );
|
|
$lnk = $t ? $skin->makeLinkObj( $t, wfMsgHTML("edit"), 'action=edit' ) : htmlspecialchars($section);
|
|
$ttext = wfMsgExt( "gadget-section-$section", $msgOpt );
|
|
|
|
if( $listOpen ) {
|
|
$wgOut->addHTML( '</ul>' );
|
|
$listOpen = false;
|
|
}
|
|
$wgOut->addHTML( "\n<h2>$ttext [$lnk]</h2>\n" );
|
|
}
|
|
|
|
foreach ( $entries as $gname => $code ) {
|
|
$t = Title::makeTitleSafe( NS_MEDIAWIKI, "Gadget-$gname$lang" );
|
|
if ( !$t ) continue;
|
|
|
|
$lnk = $skin->makeLinkObj( $t, wfMsgHTML("edit"), 'action=edit' );
|
|
$ttext = wfMsgExt( "gadget-$gname", $msgOpt );
|
|
|
|
if( !$listOpen ) {
|
|
$listOpen = true;
|
|
$wgOut->addHTML( '<ul>' );
|
|
}
|
|
$wgOut->addHTML( "<li>" );
|
|
$wgOut->addHTML( "$ttext [$lnk]<br />" );
|
|
|
|
$wgOut->addHTML( wfMsgHTML( "gadgets-uses" ) . wfMsg( 'colon-separator' ) );
|
|
|
|
$first = true;
|
|
foreach ( $code as $codePage ) {
|
|
$t = Title::makeTitleSafe( NS_MEDIAWIKI, "Gadget-$codePage" );
|
|
if ( !$t ) continue;
|
|
|
|
if ( $first ) $first = false;
|
|
else $wgOut->addHTML(", ");
|
|
|
|
$lnk = $skin->makeLinkObj( $t, htmlspecialchars( $t->getText() ) );
|
|
$wgOut->addHTML($lnk);
|
|
}
|
|
|
|
$wgOut->addHTML( "</li>" );
|
|
}
|
|
}
|
|
|
|
if( $listOpen ) {
|
|
$wgOut->addHTML( '</ul>' );
|
|
}
|
|
}
|
|
}
|