mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-14 19:28:31 +00:00
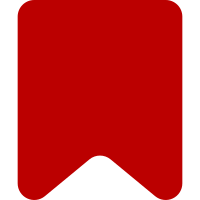
* DB changes - Create a table for push topics NameTableStore - Add a foreign key on subscriptions to normalize push topics * Implement NameTableStore to normalize topics * Update DB query joins to include topic from foreignkey * Adapt code to use IDs instead of the actual topic Bug: T261756 Change-Id: Ia7589f4a607993352d45b2dd3bcb3867d12d6e97
42 lines
1.4 KiB
PHP
42 lines
1.4 KiB
PHP
<?php
|
|
|
|
use EchoPush\Subscription;
|
|
use Wikimedia\Timestamp\ConvertibleTimestamp;
|
|
|
|
/** @covers \EchoPush\Subscription */
|
|
class SubscriptionTest extends MediaWikiUnitTestCase {
|
|
|
|
public function testNewFromRow(): void {
|
|
$row = new stdClass();
|
|
$row->eps_token = 'AABC123';
|
|
$row->epp_name = 'fcm';
|
|
$row->eps_data = null;
|
|
$row->ept_text = null;
|
|
$row->eps_updated = '2020-01-01 10:10:10';
|
|
|
|
$subscription = Subscription::newFromRow( $row );
|
|
$this->assertSame( 'AABC123', $subscription->getToken() );
|
|
$this->assertSame( 'fcm', $subscription->getProvider() );
|
|
$this->assertNull( $subscription->getTopic() );
|
|
$this->assertInstanceOf( ConvertibleTimestamp::class, $subscription->getUpdated() );
|
|
$this->assertSame( '1577873410', $subscription->getUpdated()->getTimestamp() );
|
|
}
|
|
|
|
public function testNewFromRowWithTopic(): void {
|
|
$row = new stdClass();
|
|
$row->eps_token = 'DEF456';
|
|
$row->epp_name = 'apns';
|
|
$row->eps_data = null;
|
|
$row->ept_text = 'test';
|
|
$row->eps_updated = '2020-01-01 10:10:10';
|
|
|
|
$subscription = Subscription::newFromRow( $row );
|
|
$this->assertSame( 'DEF456', $subscription->getToken() );
|
|
$this->assertSame( 'apns', $subscription->getProvider() );
|
|
$this->assertSame( 'test', $subscription->getTopic() );
|
|
$this->assertInstanceOf( ConvertibleTimestamp::class, $subscription->getUpdated() );
|
|
$this->assertSame( '1577873410', $subscription->getUpdated()->getTimestamp() );
|
|
}
|
|
|
|
}
|