mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-14 19:28:31 +00:00
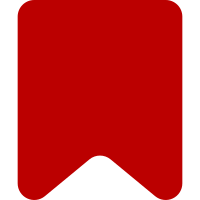
* mediawiki/mediawiki-codesniffer: 35.0.0 → 36.0.0 * php-parallel-lint/php-parallel-lint: 1.2.0 → 1.3.0 Change-Id: I4b2fb7f46b6c0a5c33a6ad25f085de8ae13eb084
87 lines
1.9 KiB
PHP
87 lines
1.9 KiB
PHP
<?php
|
|
|
|
use EchoPush\NotificationServiceClient;
|
|
use EchoPush\Subscription;
|
|
|
|
/** @covers \EchoPush\NotificationServiceClient */
|
|
class NotificationServiceClientUnitTest extends MediaWikiUnitTestCase {
|
|
|
|
/**
|
|
* @dataProvider sendCheckEchoRequestsProvider
|
|
*/
|
|
public function testSendCheckEchoRequests( $numOfCalls, $subscriptions, $expected ): void {
|
|
$mock = $this->getMockBuilder( NotificationServiceClient::class )
|
|
->disableOriginalConstructor()
|
|
->onlyMethods( [ 'sendRequest' ] )
|
|
->getMock();
|
|
|
|
$mock->expects( $this->exactly( $numOfCalls ) )
|
|
->method( 'sendRequest' )
|
|
->withConsecutive( ...$expected );
|
|
|
|
$mock->sendCheckEchoRequests( $subscriptions );
|
|
}
|
|
|
|
public function sendCheckEchoRequestsProvider(): array {
|
|
$row = new stdClass();
|
|
$row->eps_token = 'JKL123';
|
|
$row->epp_name = 'fcm';
|
|
$row->eps_data = null;
|
|
$row->ept_text = null;
|
|
$row->eps_updated = '2020-01-01 10:10:10';
|
|
$subscriptions[] = Subscription::newFromRow( $row );
|
|
|
|
$row->eps_token = 'DEF456';
|
|
$row->epp_name = 'fcm';
|
|
$row->eps_data = null;
|
|
$row->ept_text = null;
|
|
$row->eps_updated = '2020-01-01 10:10:10';
|
|
$subscriptions[] = Subscription::newFromRow( $row );
|
|
|
|
$row->eps_token = 'GHI789';
|
|
$row->epp_name = 'apns';
|
|
$row->eps_data = null;
|
|
$row->ept_text = 'test';
|
|
$row->eps_updated = '2020-01-01 10:10:10';
|
|
$subscriptions[] = Subscription::newFromRow( $row );
|
|
|
|
return [
|
|
[
|
|
1,
|
|
[ $subscriptions[0], $subscriptions[1] ],
|
|
[
|
|
[
|
|
'fcm',
|
|
[
|
|
'deviceTokens' => [ "JKL123", 'DEF456' ],
|
|
'messageType' => 'checkEchoV1'
|
|
]
|
|
]
|
|
]
|
|
],
|
|
[
|
|
2,
|
|
$subscriptions,
|
|
[
|
|
[
|
|
'fcm',
|
|
[
|
|
'deviceTokens' => [ "JKL123", 'DEF456' ],
|
|
'messageType' => 'checkEchoV1'
|
|
]
|
|
],
|
|
[
|
|
'apns',
|
|
[
|
|
'deviceTokens' => [ 'GHI789' ],
|
|
'messageType' => 'checkEchoV1',
|
|
'topic' => 'test'
|
|
]
|
|
]
|
|
]
|
|
]
|
|
];
|
|
}
|
|
|
|
}
|