mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-24 10:49:37 +00:00
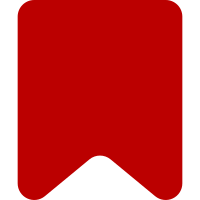
This patch makes Echo talk page notification mimic the existing Orange Bar and Email talk page notification for minor edit. For the Orange Bar, minor edit notification is sent if the editor does not have nominornewtalk permission. There are additional rules for the email, minor edit notification is sent if global $wgEnotifMinorEdit is true and notification recipient has enotifminoredits option on. Change-Id: Ib3835c4dd57a3686b227c44710a14ab06cded166
158 lines
5.6 KiB
PHP
158 lines
5.6 KiB
PHP
<?php
|
|
|
|
// @todo Fill in
|
|
class EchoNotifier {
|
|
/**
|
|
* Record an EchoNotification for an EchoEvent
|
|
* Currently used for web-based notifications.
|
|
*
|
|
* @param $user User to notify.
|
|
* @param $event EchoEvent to notify about.
|
|
*/
|
|
public static function notifyWithNotification( $user, $event ) {
|
|
global $wgEchoConfig, $wgEchoNotifications;
|
|
|
|
// Only create the notification if the user wants to recieve that type
|
|
// of notification and they are eligible to recieve it. See bug 47664.
|
|
$userWebNotifications = EchoNotificationController::getUserEnabledEvents( $user, 'web' );
|
|
if ( !in_array( $event->getType(), $userWebNotifications ) ) {
|
|
return;
|
|
}
|
|
|
|
EchoNotification::create( array( 'user' => $user, 'event' => $event ) );
|
|
|
|
self::logEvent( $user, $event, 'web' );
|
|
|
|
EchoNotificationController::resetNotificationCount( $user, DB_MASTER );
|
|
}
|
|
|
|
/**
|
|
* Store Event Logging data for web or email notifications
|
|
*
|
|
* @param $user User being notified.
|
|
* @param $event EchoEvent to log detail about.
|
|
* @param $deliveryMethod string containing either 'web' or 'email'
|
|
*/
|
|
public static function logEvent( $user, $event, $deliveryMethod ) {
|
|
global $wgEchoConfig, $wgEchoNotifications;
|
|
if ( !$wgEchoConfig['eventlogging']['Echo']['enabled'] ) {
|
|
// Only attempt event logging if Echo schema is enabled
|
|
return;
|
|
}
|
|
|
|
$agent = $event->getAgent();
|
|
// Typically an event should always have an agent, but agent could be
|
|
// null if the data is corrupted
|
|
if ( $agent ) {
|
|
$sender = $agent->isAnon() ? $agent->getName() : $agent->getId();
|
|
} else {
|
|
$sender = -1;
|
|
}
|
|
|
|
if ( isset( $wgEchoNotifications[$event->getType()]['group'] ) ) {
|
|
$group = $wgEchoNotifications[$event->getType()]['group'];
|
|
} else {
|
|
$group = 'neutral';
|
|
}
|
|
$data = array (
|
|
'version' => $wgEchoConfig['version'],
|
|
'eventId' => $event->getId(),
|
|
'notificationType' => $event->getType(),
|
|
'notificationGroup' => $group,
|
|
'sender' => (string)$sender,
|
|
'recipientUserId' => $user->getId(),
|
|
'recipientEditCount' => (int)$user->getEditCount()
|
|
);
|
|
// Add the source if it exists. (This is mostly for the Thanks extension.)
|
|
$extra = $event->getExtra();
|
|
if ( isset( $extra['source'] ) ) {
|
|
$data['eventSource'] = (string)$extra['source'];
|
|
}
|
|
if( $deliveryMethod == 'email' ) {
|
|
$data['deliveryMethod'] = 'email';
|
|
} else {
|
|
// whitelist valid delivery methods so it is always valid
|
|
$data['deliveryMethod'] = 'web';
|
|
}
|
|
EchoHooks::logEvent( 'Echo', $data );
|
|
}
|
|
|
|
/**
|
|
* Send a Notification to a user by email
|
|
*
|
|
* @param $user User to notify.
|
|
* @param $event EchoEvent to notify about.
|
|
* @return bool
|
|
*/
|
|
public static function notifyWithEmail( $user, $event ) {
|
|
// No valid email address or email notification
|
|
if ( !$user->isEmailConfirmed() || $user->getOption( 'echo-email-frequency' ) < 0 ) {
|
|
return false;
|
|
}
|
|
|
|
// Final check on whether to send email for this user & event
|
|
if ( !wfRunHooks( 'EchoAbortEmailNotification', array( $user, $event ) ) ) {
|
|
return false;
|
|
}
|
|
|
|
// See if the user wants to receive emails for this category or the user is eligible to receive this email
|
|
if ( in_array( $event->getType(), EchoNotificationController::getUserEnabledEvents( $user, 'email' ) ) ) {
|
|
global $wgEchoEnableEmailBatch, $wgEchoNotifications, $wgNotificationSender, $wgNotificationSenderName, $wgNotificationReplyName, $wgEchoBundleEmailInterval;
|
|
|
|
$priority = EchoNotificationController::getNotificationPriority( $event->getType() );
|
|
|
|
$bundleString = $bundleHash = '';
|
|
|
|
// We should have bundling for email digest as long as either web or email bundling is on, for example, talk page
|
|
// email bundling is off, but if a user decides to receive email digest, we should bundle those messages
|
|
if ( !empty( $wgEchoNotifications[$event->getType()]['bundle']['web'] ) || !empty( $wgEchoNotifications[$event->getType()]['bundle']['email'] ) ) {
|
|
wfRunHooks( 'EchoGetBundleRules', array( $event, &$bundleString ) );
|
|
}
|
|
if ( $bundleString ) {
|
|
$bundleHash = md5( $bundleString );
|
|
}
|
|
|
|
self::logEvent( $user, $event, 'email' );
|
|
|
|
// email digest notification ( weekly or daily )
|
|
if ( $wgEchoEnableEmailBatch && $user->getOption( 'echo-email-frequency' ) > 0 ) {
|
|
// always create a unique event hash for those events don't support bundling
|
|
// this is mainly for group by
|
|
if ( !$bundleHash ) {
|
|
$bundleHash = md5( $event->getType() . '-' . $event->getId() );
|
|
}
|
|
MWEchoEmailBatch::addToQueue( $user->getId(), $event->getId(), $priority, $bundleHash );
|
|
return true;
|
|
}
|
|
|
|
$addedToQueue = false;
|
|
|
|
// only send bundle email if email bundling is on
|
|
if ( $wgEchoBundleEmailInterval && $bundleHash && !empty( $wgEchoNotifications[$event->getType()]['bundle']['email'] ) ) {
|
|
$bundler = MWEchoEmailBundler::newFromUserHash( $user, $bundleHash );
|
|
if ( $bundler ) {
|
|
$addedToQueue = $bundler->addToEmailBatch( $event->getId(), $priority );
|
|
}
|
|
}
|
|
|
|
// send single notification if the email wasn't added to queue for bundling
|
|
if ( !$addedToQueue ) {
|
|
// instant email notification
|
|
$toAddress = new MailAddress( $user );
|
|
$fromAddress = new MailAddress( $wgNotificationSender, $wgNotificationSenderName );
|
|
$replyAddress = new MailAddress( $wgNotificationSender, $wgNotificationReplyName );
|
|
// Since we are sending a single email, should set the bundle hash to null
|
|
// if it is set with a value from somewhere else
|
|
$event->setBundleHash( null );
|
|
$email = EchoNotificationController::formatNotification( $event, $user, 'email', 'email' );
|
|
$subject = $email['subject'];
|
|
$body = $email['body'];
|
|
|
|
UserMailer::send( $toAddress, $fromAddress, $subject, $body, $replyAddress );
|
|
}
|
|
}
|
|
|
|
return true;
|
|
}
|
|
}
|