mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
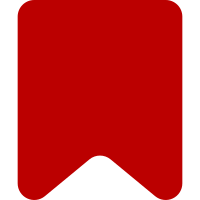
This should have been done from the beginning; the model manager pulls models by their symbolic names. So far, we've used the source for that, but that assumes that two modules always have different sources, and that is absolutely not necessarily the case. For example, internal local bundles will each be a model, but have the same ('local') source. They should still be differentiated in the manager by their names, but the source should state clearly that it is local. For this, the models now have "getName" method and the name is created separately from their source. Items also preserve a reference to their parent's symbolic name so they can provide that for items that require the controller to manipulate a specific model. Change-Id: I8c39d5d28383d11fb330addce21e07d5c424da6f
132 lines
3.3 KiB
JavaScript
132 lines
3.3 KiB
JavaScript
( function ( mw ) {
|
|
/**
|
|
* Cross-wiki notification item model. Contains a list of sources,
|
|
* that each contain a list of notification items from that source.
|
|
*
|
|
* @class
|
|
* @extends mw.echo.dm.NotificationItem
|
|
*
|
|
* @constructor
|
|
* @param {number} id Notification id
|
|
* @param {Object} [config] Configuration object
|
|
* @cfg {number} count The initial anticipated count of notifications through all
|
|
* of the sources.
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem = function MwEchoDmCrossWikiNotificationItem( id, config ) {
|
|
config = config || {};
|
|
|
|
mw.echo.dm.CrossWikiNotificationItem.parent.call( this, id, config );
|
|
|
|
this.foreign = true;
|
|
this.count = config.count || 0;
|
|
|
|
this.list = new mw.echo.dm.NotificationGroupsList();
|
|
|
|
this.list.connect( this, { remove: 'onListRemove' } );
|
|
};
|
|
|
|
OO.inheritClass( mw.echo.dm.CrossWikiNotificationItem, mw.echo.dm.NotificationItem );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event removeSource
|
|
* @param {string} name The symbolic name for the source that was removed
|
|
*
|
|
* Source list has been removed
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Respond to list being removed from the cross-wiki bundle.
|
|
*
|
|
* @param {mw.echo.dm.NotificationGroupsList} sourceModel The source model that was removed
|
|
* @fires removeSource
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.onListRemove = function ( sourceModel ) {
|
|
this.emit( 'removeSource', sourceModel.getName() );
|
|
|
|
};
|
|
|
|
/**
|
|
* Get the list of sources
|
|
*
|
|
* @return {mw.echo.dm.NotificationGroupsList} List of sources
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.getList = function () {
|
|
return this.list;
|
|
};
|
|
|
|
/**
|
|
* Get an array of source names that are in the cross-wiki list
|
|
*
|
|
* @return {string[]} Source names
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.getSourceNames = function () {
|
|
var i,
|
|
sourceNames = [],
|
|
sourceLists = this.list.getItems();
|
|
|
|
for ( i = 0; i < sourceLists.length; i++ ) {
|
|
sourceNames.push( sourceLists[ i ].getName() );
|
|
}
|
|
|
|
return sourceNames;
|
|
};
|
|
|
|
/**
|
|
* Get a specific item from the list by its source name
|
|
*
|
|
* @param {string} sourceName Source name
|
|
* @return {mw.echo.dm.NotificationGroupsList} Source item
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.getItemBySource = function ( sourceName ) {
|
|
return this.list.getGroupBySource( sourceName );
|
|
};
|
|
|
|
/**
|
|
* Get expected item count from all sources
|
|
*
|
|
* @return {number} Item count
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.getCount = function () {
|
|
return this.count;
|
|
};
|
|
|
|
/**
|
|
* Check if there are unseen items in any of the cross wiki source lists.
|
|
* This method is required for all models that are managed by the
|
|
* mw.echo.dm.ModelManager.
|
|
*
|
|
* @return {boolean} There are unseen items
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.hasUnseen = function () {
|
|
var i, j, items,
|
|
sourceLists = this.getList().getItems();
|
|
|
|
for ( i = 0; i < sourceLists.length; i++ ) {
|
|
items = sourceLists[ i ].getItems();
|
|
for ( j = 0; j < items.length; j++ ) {
|
|
if ( !items[ j ].isSeen() ) {
|
|
return true;
|
|
}
|
|
}
|
|
}
|
|
|
|
return false;
|
|
};
|
|
|
|
/**
|
|
* This item is a group.
|
|
* This method is required for all models that are managed by the
|
|
* mw.echo.dm.ModelManager.
|
|
*
|
|
* @return {boolean} This item is a group
|
|
*/
|
|
mw.echo.dm.CrossWikiNotificationItem.prototype.isGroup = function () {
|
|
return true;
|
|
};
|
|
|
|
} )( mediaWiki );
|