mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-23 18:30:06 +00:00
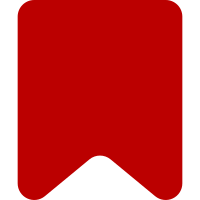
Keep the rule and prefer-const disabled as they will require manual fixing. Change-Id: Idd31eb7d7a456b10c07b905b4b837dd4c890eb71
65 lines
2.1 KiB
JavaScript
65 lines
2.1 KiB
JavaScript
( function () {
|
|
/* global moment:false */
|
|
/**
|
|
* A sub group widget that displays notifications divided by dates.
|
|
*
|
|
* @class
|
|
* @extends mw.echo.ui.SubGroupListWidget
|
|
*
|
|
* @constructor
|
|
* @param {mw.echo.Controller} controller Notifications controller
|
|
* @param {mw.echo.dm.SortedList} listModel Notifications list model for this source
|
|
* @param {Object} [config] Configuration object
|
|
*/
|
|
mw.echo.ui.DatedSubGroupListWidget = function MwEchoUiDatedSubGroupListWidget( controller, listModel, config ) {
|
|
const now = moment(),
|
|
$primaryDate = $( '<span>' )
|
|
.addClass( 'mw-echo-ui-datedSubGroupListWidget-title-primary' ),
|
|
$secondaryDate = $( '<span>' )
|
|
.addClass( 'mw-echo-ui-datedSubGroupListWidget-title-secondary' ),
|
|
$title = $( '<h2>' )
|
|
.addClass( 'mw-echo-ui-datedSubGroupListWidget-title' )
|
|
.append( $primaryDate, $secondaryDate );
|
|
|
|
// Parent constructor
|
|
mw.echo.ui.DatedSubGroupListWidget.super.call( this, controller, listModel, $.extend( {
|
|
// Since this widget is defined as a dated list, we sort
|
|
// its items according to timestamp without consideration
|
|
// of read state or foreignness.
|
|
sortingCallback: function ( a, b ) {
|
|
// Reverse sorting
|
|
if ( b.getTimestamp() < a.getTimestamp() ) {
|
|
return -1;
|
|
} else if ( b.getTimestamp() > a.getTimestamp() ) {
|
|
return 1;
|
|
}
|
|
|
|
// Fallback on IDs
|
|
return b.getId() - a.getId();
|
|
}
|
|
}, config ) );
|
|
|
|
// Round all dates to the day they're in, as if they all happened at 00:00h
|
|
const stringTimestamp = moment.utc( this.model.getTimestamp() ).local().format( 'YYYY-MM-DD' );
|
|
const momentTimestamp = moment( stringTimestamp );
|
|
const diff = now.diff( momentTimestamp, 'weeks' );
|
|
const fullDate = momentTimestamp.format( 'LL' );
|
|
|
|
$primaryDate.text( fullDate );
|
|
if ( diff === 0 ) {
|
|
$secondaryDate.text( fullDate );
|
|
momentTimestamp.locale( 'echo-shortRelativeTime' );
|
|
$primaryDate.text( momentTimestamp.calendar() );
|
|
}
|
|
|
|
this.title.setLabel( $title );
|
|
|
|
this.$element
|
|
.addClass( 'mw-echo-ui-datedSubGroupListWidget' );
|
|
};
|
|
|
|
/* Initialization */
|
|
|
|
OO.inheritClass( mw.echo.ui.DatedSubGroupListWidget, mw.echo.ui.SubGroupListWidget );
|
|
}() );
|