mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
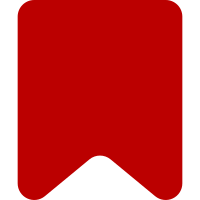
In addition, any message used in the email should be sent in the user's language. Bug: 52992 Change-Id: I3585f28d4ec97b86a467958bdfd603791f293d52
65 lines
1.7 KiB
PHP
65 lines
1.7 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Formatter for 'user-rights' notifications
|
|
*/
|
|
class EchoUserRightsFormatter extends EchoBasicFormatter {
|
|
|
|
/**
|
|
* @param $event EchoEvent
|
|
* @param $param string
|
|
* @param $message Message
|
|
* @param $user User
|
|
*/
|
|
protected function processParam( $event, $param, $message, $user ) {
|
|
$extra = $event->getExtra();
|
|
|
|
switch ( $param ) {
|
|
// List of user rights that are granted or revoked
|
|
case 'user-rights-list':
|
|
global $wgLang;
|
|
|
|
$list = array();
|
|
|
|
foreach ( array( 'add', 'remove' ) as $action ) {
|
|
if ( isset( $extra[$action] ) && $extra[$action] ) {
|
|
// Messages that can be used here:
|
|
// * notification-user-rights-add
|
|
// * notification-user-rights-remove
|
|
$list[] = $this->getMessage( 'notification-user-rights-' . $action )
|
|
->params( $wgLang->commaList( $extra[$action] ), count( $extra[$action] ) )
|
|
->escaped();
|
|
}
|
|
}
|
|
$message->params( $wgLang->semicolonList( $list ) );
|
|
break;
|
|
|
|
default:
|
|
parent::processParam( $event, $param, $message, $user );
|
|
break;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Helper function for getLink()
|
|
*
|
|
* @param EchoEvent $event
|
|
* @param User $user The user receiving the notification
|
|
* @param String $destination The destination type for the link
|
|
* @return Array including target and query parameters
|
|
*/
|
|
protected function getLinkParams( $event, $user, $destination ) {
|
|
$target = null;
|
|
$query = array();
|
|
// Set up link parameters based on the destination (or pass to parent)
|
|
switch ( $destination ) {
|
|
case 'user-rights-list':
|
|
$target = SpecialPage::getTitleFor( 'Listgrouprights' );
|
|
break;
|
|
default:
|
|
return parent::getLinkParams( $event, $user, $destination );
|
|
}
|
|
return array( $target, $query );
|
|
}
|
|
}
|