mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 11:17:49 +00:00
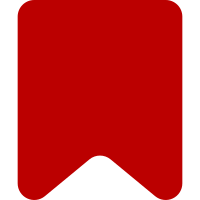
Avoid duplicate by looking if the notification already exist. Change-Id: Ie519d13cd0ab03919f70da90d58c82a214c74b49 Depends-On: I79194c41d6b2fd84ad658909a2941d9d3d28d94e Bug: T128249
59 lines
1.8 KiB
PHP
59 lines
1.8 KiB
PHP
<?php
|
|
|
|
/**
|
|
* @group Echo
|
|
*/
|
|
class MWEchoThankYouEditTest extends MediaWikiTestCase {
|
|
|
|
protected function setUp() {
|
|
parent::setUp();
|
|
}
|
|
|
|
public function testFirstEdit() {
|
|
// setup
|
|
$user = $this->getMutableTestUser()->getUser();
|
|
$title = Title::newFromText( 'Help:MWEchoThankYouEditTest_testFirstEdit' );
|
|
|
|
// action
|
|
$this->edit( $title, $user, 'this is my first edit' );
|
|
|
|
// assertions
|
|
$notificationMapper = new EchoNotificationMapper();
|
|
$notifications = $notificationMapper->fetchByUser( $user, 10, null, array( 'thank-you-edit' ) );
|
|
$this->assertCount( 1, $notifications );
|
|
|
|
/** @var EchoNotification $notification */
|
|
$notification = reset( $notifications );
|
|
$this->assertEquals( 1, $notification->getEvent()->getExtraParam( 'editCount', 'not found' ) );
|
|
}
|
|
|
|
public function testTenthEdit() {
|
|
// setup
|
|
$user = $this->getMutableTestUser()->getUser();
|
|
$title = Title::newFromText( 'Help:MWEchoThankYouEditTest_testTenthEdit' );
|
|
|
|
// action
|
|
// we could fast-forward the edit-count to speed things up
|
|
// but this is the only way to make sure duplicate notifications
|
|
// are not generated
|
|
for ( $i = 0; $i < 12; $i++ ) {
|
|
$this->edit( $title, $user, "this is edit #$i" );
|
|
}
|
|
|
|
// assertions
|
|
$notificationMapper = new EchoNotificationMapper();
|
|
$notifications = $notificationMapper->fetchByUser( $user, 10, null, array( 'thank-you-edit' ) );
|
|
$this->assertCount( 2, $notifications );
|
|
|
|
/** @var EchoNotification $notification */
|
|
$notification = reset( $notifications );
|
|
$this->assertEquals( 10, $notification->getEvent()->getExtraParam( 'editCount', 'not found' ) );
|
|
}
|
|
|
|
private function edit( Title $title, User $user, $text ) {
|
|
$page = WikiPage::factory( $title );
|
|
$content = ContentHandler::makeContent( $text, $title );
|
|
$page->doEditContent( $content, 'test', 0, false, $user );
|
|
}
|
|
}
|