mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 03:09:37 +00:00
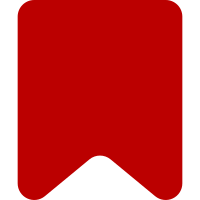
Implements whitelists and blacklists for notification agents to assist in filtering out unwanted notifications from bots. Bug: 47946 Change-Id: I0d7e071067c6974fb90cf6c0ba1bd159f46bd5df
71 lines
2.2 KiB
PHP
71 lines
2.2 KiB
PHP
<?php
|
|
|
|
class ContainmentSetTest extends MediaWikiTestCase {
|
|
|
|
public function testGenericContains() {
|
|
$list = new EchoContainmentSet;
|
|
|
|
$list->addArray( array( 'foo', 'bar' ) );
|
|
$this->assertTrue( $list->contains( 'foo' ) );
|
|
$this->assertTrue( $list->contains( 'bar' ) );
|
|
$this->assertFalse( $list->contains( 'whammo' ) );
|
|
|
|
$list->addArray( array( 'whammo' ) );
|
|
$this->assertTrue( $list->contains( 'whammo' ) );
|
|
}
|
|
|
|
public function testCachedListInnerListIsOnlyCalledOnce() {
|
|
|
|
// the global $wgMemc during tests is an EmptyBagOStuff, so it
|
|
// wont do anything. We use a HashBagOStuff to get more like a real
|
|
// client
|
|
$innerCache = new HashBagOStuff;
|
|
|
|
$inner = array( 'bing', 'bang' );
|
|
// We use a mock instead of the real thing for the $this->once() assertion
|
|
// verifying that the cache doesn't just keep asking the inner object
|
|
$list = $this->getMockBuilder('EchoArrayList')
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$list->expects( $this->once() )
|
|
->method( 'getValues' )
|
|
->will( $this->returnValue( $inner ) );
|
|
|
|
$cached = new EchoCachedList( $innerCache, 'test_key', $list );
|
|
|
|
// First run through should hit the main list, and save to innerCache
|
|
$this->assertEquals( $inner, $cached->getValues() );
|
|
$this->assertEquals( $inner, $cached->getValues() );
|
|
|
|
// Reinitialize to get a fresh instance that will pull directly from
|
|
// innerCache without hitting the $list
|
|
$freshCached = new EchoCachedList( $innerCache, 'test_key', $list );
|
|
$this->assertEquals( $inner, $freshCached->getValues() );
|
|
}
|
|
|
|
/**
|
|
* @Database
|
|
*/
|
|
public function testOnWikiList() {
|
|
$this->editPage( 'User:Foo/Bar-baz', "abc\ndef\r\nghi\n\n\n" );
|
|
|
|
$list = new EchoOnWikiList( NS_USER, "Foo/Bar-baz" );
|
|
$this->assertEquals(
|
|
array( 'abc', 'def', 'ghi' ),
|
|
$list->getValues()
|
|
);
|
|
}
|
|
|
|
public function testOnWikiListNonExistant() {
|
|
$list = new EchoOnWikiList( NS_USER, "Some_Non_Existant_Page" );
|
|
$this->assertEquals( array(), $list->getValues() );
|
|
}
|
|
|
|
protected function editPage( $pageName, $text, $summary = '', $defaultNs = NS_MAIN ) {
|
|
$title = Title::newFromText( $pageName, $defaultNs );
|
|
$page = WikiPage::factory( $title );
|
|
|
|
return $page->doEditContent( ContentHandler::makeContent( $text, $title ), $summary );
|
|
}
|
|
}
|