mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-24 16:04:35 +00:00
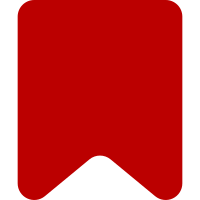
We used to have to DIY it because the core method stripped links, but with guessSectionNameFromStrippedText() this is no longer an issue. This allows us to pick up the nbsp handling that was added to core in 129067c907. Bug: T180689 Depends-On: I56b9dda805a51517549c5ed709f4bd747ca04577 Change-Id: I192218dd14464de5041ceb1c16125bbcd8f44f18
93 lines
2.3 KiB
PHP
93 lines
2.3 KiB
PHP
<?php
|
|
/**
|
|
* Trait that adds section title handling to an EchoEventPresentationModel subclass.
|
|
*/
|
|
trait EchoPresentationModelSectionTrait {
|
|
private $rawSectionTitle = null;
|
|
private $parsedSectionTitle = null;
|
|
|
|
/**
|
|
* Get the raw (unparsed) section title
|
|
* @return string Section title
|
|
*/
|
|
protected function getRawSectionTitle() {
|
|
if ( $this->rawSectionTitle !== null ) {
|
|
return $this->rawSectionTitle;
|
|
}
|
|
$sectionTitle = $this->event->getExtraParam( 'section-title' );
|
|
if ( !$sectionTitle ) {
|
|
$this->rawSectionTitle = false;
|
|
return false;
|
|
}
|
|
// Check permissions
|
|
if ( !$this->userCan( Revision::DELETED_TEXT ) ) {
|
|
$this->rawSectionTitle = false;
|
|
return false;
|
|
}
|
|
|
|
$this->rawSectionTitle = $sectionTitle;
|
|
return $this->rawSectionTitle;
|
|
}
|
|
|
|
/**
|
|
* Get the section title parsed to plain text
|
|
* @return string Section title (plain text)
|
|
*/
|
|
protected function getParsedSectionTitle() {
|
|
if ( $this->parsedSectionTitle !== null ) {
|
|
return $this->parsedSectionTitle;
|
|
}
|
|
$rawSectionTitle = $this->getRawSectionTitle();
|
|
if ( !$rawSectionTitle ) {
|
|
$this->parsedSectionTitle = false;
|
|
return false;
|
|
}
|
|
$this->parsedSectionTitle = EchoDiscussionParser::getTextSnippet(
|
|
$rawSectionTitle,
|
|
$this->language,
|
|
150,
|
|
$this->event->getTitle()
|
|
);
|
|
return $this->parsedSectionTitle;
|
|
}
|
|
|
|
/**
|
|
* Check if there is a section.
|
|
*
|
|
* This also returns false if the revision is deleted,
|
|
* even if there is a section, because the section can't
|
|
* be viewed in that case.
|
|
* @return bool Whether there is a section
|
|
*/
|
|
protected function hasSection() {
|
|
return (bool)$this->getRawSectionTitle();
|
|
}
|
|
|
|
/**
|
|
* Get a Title pointing to the section, if available.
|
|
* @return Title
|
|
*/
|
|
protected function getTitleWithSection() {
|
|
$title = $this->event->getTitle();
|
|
$section = $this->getParsedSectionTitle();
|
|
$fragment = substr( Parser::guessSectionNameFromStrippedText( $section ), 1 );
|
|
if ( $section ) {
|
|
$title = Title::makeTitle(
|
|
$title->getNamespace(),
|
|
$title->getDBkey(),
|
|
$fragment
|
|
);
|
|
}
|
|
return $title;
|
|
}
|
|
|
|
protected function getTruncatedSectionTitle() {
|
|
return $this->language->embedBidi( $this->language->truncate(
|
|
$this->getParsedSectionTitle(),
|
|
self::SECTION_TITLE_RECOMMENDED_LENGTH,
|
|
'...',
|
|
false
|
|
) );
|
|
}
|
|
}
|