mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-13 17:57:21 +00:00
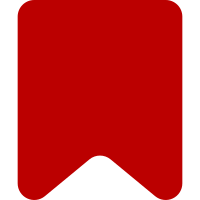
Per https://www.mediawiki.org/wiki/CC/JS#Linting. Update a few files to bring the tests closer to passing. Change-Id: I08b190e8b44dbdbeb56de5c380d0ed4a8394aca2
170 lines
4.7 KiB
JavaScript
170 lines
4.7 KiB
JavaScript
( function ( $, mw ) {
|
|
/*global alert */
|
|
'use strict';
|
|
|
|
mw.echo = {
|
|
|
|
'optionsToken': '',
|
|
|
|
'dismissOutputFormats': ['web', 'email'],
|
|
|
|
/**
|
|
* Change the user's preferences related to this notification type and
|
|
* reload the page.
|
|
*/
|
|
'dismiss': function( notification ) {
|
|
var $notification = $( notification ),
|
|
eventCategory = $notification.attr( 'data-notification-category' ),
|
|
prefName = '',
|
|
prefs = [],
|
|
prefRequest = {};
|
|
$.each( mw.echo.dismissOutputFormats, function( index, format ) {
|
|
// Make sure output format pref exists for this event type
|
|
prefName = 'echo-subscriptions-' + format + '-' + eventCategory;
|
|
if ( mw.user.options.exists( prefName ) ) {
|
|
prefs.push( prefName + '=0' );
|
|
}
|
|
} );
|
|
prefRequest = {
|
|
action: 'options',
|
|
change: prefs.join( '|' ),
|
|
token: mw.echo.optionsToken,
|
|
format: 'json'
|
|
};
|
|
$.ajax( {
|
|
type: 'post',
|
|
url: mw.util.wikiScript( 'api' ),
|
|
data: prefRequest,
|
|
dataType: 'json',
|
|
success: function () {
|
|
// If we're on the Notifications archive page, just refresh the page
|
|
if ( mw.config.get( 'wgCanonicalNamespace' ) === 'Special' &&
|
|
mw.config.get( 'wgCanonicalSpecialPageName' ) === 'Notifications'
|
|
) {
|
|
window.location.reload();
|
|
} else {
|
|
eventCategory = $notification.attr( 'data-notification-category' );
|
|
$( '.mw-echo-overlay li[data-notification-category="' + eventCategory + '"]' ).hide();
|
|
$notification.data( 'dismiss', false );
|
|
}
|
|
},
|
|
error: function() {
|
|
alert( mw.msg( 'echo-error-preference' ) );
|
|
}
|
|
} );
|
|
},
|
|
|
|
/**
|
|
* Handle clicking the Dismiss button.
|
|
* First we have to retrieve the options token.
|
|
*/
|
|
'setOptionsToken': function( callback, notification ) {
|
|
var tokenRequest,
|
|
_this = this;
|
|
|
|
tokenRequest = {
|
|
'action': 'tokens',
|
|
'type' : 'options',
|
|
'format': 'json'
|
|
};
|
|
if ( this.optionsToken ) {
|
|
callback( notification );
|
|
} else {
|
|
$.ajax( {
|
|
type: 'get',
|
|
url: mw.util.wikiScript( 'api' ),
|
|
data: tokenRequest,
|
|
dataType: 'json',
|
|
success: function( data ) {
|
|
if ( data.tokens.optionstoken === undefined ) {
|
|
alert( mw.msg( 'echo-error-token' ) );
|
|
} else {
|
|
_this.optionsToken = data.tokens.optionstoken;
|
|
callback( notification );
|
|
}
|
|
},
|
|
error: function() {
|
|
alert( mw.msg( 'echo-error-token' ) );
|
|
}
|
|
} );
|
|
}
|
|
},
|
|
|
|
/**
|
|
* Show the dismiss interface (Dismiss and Cancel buttons).
|
|
*/
|
|
'showDismissOption': function( closeBox ) {
|
|
var $notification = $( closeBox ).parent();
|
|
$( closeBox ).hide();
|
|
$notification.data( 'dismiss', true );
|
|
$notification.height( $notification.find( '.mw-echo-dismiss' ).height() - 10 );
|
|
$notification.find( '.mw-echo-dismiss' )
|
|
// Make sure the dismiss interface exactly covers the notification
|
|
// Icon adds 45px to the notification
|
|
.width( $notification.width() - 45 )
|
|
.show();
|
|
// Temprorarily ungrey-out read notifications
|
|
if ( !$notification.hasClass( 'mw-echo-unread' ) ) {
|
|
$notification.find( '.mw-echo-state' ).css( 'filter', 'alpha(opacity=100)' );
|
|
$notification.find( '.mw-echo-state' ).css( 'opacity', '1.0' );
|
|
}
|
|
},
|
|
|
|
'setUpDismissability' : function( notification ) {
|
|
var $dismissButton,
|
|
$cancelButton,
|
|
$closebox,
|
|
_this = this,
|
|
$notification = $( notification );
|
|
|
|
// Add dismiss box
|
|
$closebox = $( '<div/>' )
|
|
.addClass( 'mw-echo-close-box' )
|
|
.css( 'display', 'none' )
|
|
.click( function() {
|
|
_this.showDismissOption( this );
|
|
} );
|
|
$notification.append( $closebox );
|
|
|
|
// Add dismiss and cancel buttons
|
|
$dismissButton = $( '<button/>' )
|
|
.text( mw.msg( 'echo-dismiss-button' ) )
|
|
.addClass( 'mw-echo-dismiss-button' )
|
|
.addClass( 'ui-button-blue' )
|
|
.button( {
|
|
icons: { primary: 'ui-icon-closethick' }
|
|
} )
|
|
.click( function () {
|
|
_this.setOptionsToken( _this.dismiss, $notification );
|
|
} );
|
|
$cancelButton = $( '<a/>' )
|
|
.text( mw.msg( 'cancel' ) )
|
|
.addClass( 'mw-echo-cancel-link' )
|
|
.click( function () {
|
|
$notification.data( 'dismiss', false );
|
|
$notification.find( '.mw-echo-dismiss' ).hide();
|
|
$notification.css( 'height', 'auto' );
|
|
$closebox.show();
|
|
} );
|
|
$notification.find( '.mw-echo-dismiss' )
|
|
.append( $dismissButton )
|
|
.append( $cancelButton );
|
|
|
|
// Make each notification hot for dismissability
|
|
$notification.hover(
|
|
function() {
|
|
if ( !$( this ).data( 'dismiss' ) ) {
|
|
$( this ).find( '.mw-echo-close-box' ).show();
|
|
}
|
|
},
|
|
function() {
|
|
if ( !$( this ).data( 'dismiss' ) ) {
|
|
$( this ).find( '.mw-echo-close-box' ).hide();
|
|
}
|
|
}
|
|
);
|
|
}
|
|
|
|
};
|
|
} )( jQuery, mediaWiki );
|