mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 11:17:49 +00:00
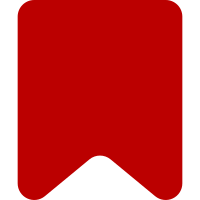
Split the notifications into 'alert' and 'message' badget with two different flyouts. Also clean up styling and module behavior. ** Depends on ooui change Id4bbe14ba0bf6c for footers in popups. ** Depends on ooui change Ie93e4d6ed5637c for fixing a bug in inverted icons. ** MobileFrontend must also be updated to support the new modules in this patch I168f485d6e54cb4067 In this change: * Split notifcations into alert and messages and display those in two different badges. * Create two separate flyout/popups for each category with their notifications. * Create a view-model to control notification state and emit events for both the popup and the badge to intercept and react to. * Clean up module load and distribution: * Create an ext.echo.ui module for javascript-ui support and ooui widgets. * Create an ext.echo.nojs module that unifies all base classes that are needed for both nojs and js support, that the js version builds upon. * Create a separate ext.echo.logger module as a singleton that can be called to perform all logging. * Clean up style uses * Move the special page LESS file into nojs module so all styles load properly even in nojs mode. * Transfer some of the styling from JS to LESS for consistency. * Make the 'read more' button load already with the styles it needs to look like a button, since its behavior is similar in nojs and js vesions, but before its classes were applied only by the js, making it inconsistent and also making its appearance 'jump' from a link to a button. * Delete and clean up all old and unused files. * Moved 'Help.png' icon from modules/overlay to modules/icons for later use. Bug: T108190 Change-Id: I55f440ed9f64c46817f620328a6bb522d44c9ca9
177 lines
4.6 KiB
JavaScript
177 lines
4.6 KiB
JavaScript
( function ( mw, $ ) {
|
|
/**
|
|
* Echo notification NotificationItem model
|
|
*
|
|
* @class
|
|
* @mixins OO.EventEmitter
|
|
*
|
|
* @constructor
|
|
* @param {number} id Notification id,
|
|
* @param {Object} [config] Configuration object
|
|
* @cfg {jQuery|string} [content] The html content of this notification
|
|
* @cfg {string} [category] The category of this notification. The category identifies
|
|
* where the notification originates from.
|
|
* @cfg {boolean} [read=false] State the read state of the option
|
|
* @cfg {boolean} [seen=false] State the seen state of the option
|
|
* @cfg {string} [timestamp] Notification timestamp in Mediawiki timestamp format
|
|
* @cfg {string} [primaryUrl] Notification primary link in raw url format
|
|
*/
|
|
mw.echo.dm.NotificationItem = function mwFlowDmNotificationItem( id, config ) {
|
|
var date = new Date(),
|
|
normalizeNumber = function ( number ) {
|
|
return ( number < 10 ? '0' : '' ) + String( number );
|
|
},
|
|
fallbackMWDate = date.getUTCFullYear() +
|
|
normalizeNumber( date.getMonth() ) +
|
|
normalizeNumber( date.getUTCDate() ) +
|
|
normalizeNumber( date.getUTCHours() ) +
|
|
normalizeNumber( date.getUTCMinutes() ) +
|
|
normalizeNumber( date.getUTCSeconds() );
|
|
|
|
// Mixin constructor
|
|
OO.EventEmitter.call( this );
|
|
|
|
this.id = id || null;
|
|
|
|
// TODO: We should work on the API to release and work with actual
|
|
// data here, rather than getting a pre-made html content of the
|
|
// notification.
|
|
this.content = config.content || $();
|
|
|
|
this.category = config.category || '';
|
|
|
|
this.toggleRead( !!config.read );
|
|
this.toggleSeen( !!config.seen );
|
|
|
|
this.setTimestamp( config.timestamp || fallbackMWDate );
|
|
this.setPrimaryUrl( config.primaryUrl );
|
|
};
|
|
|
|
/* Inheritance */
|
|
|
|
OO.mixinClass( mw.echo.dm.NotificationItem, OO.EventEmitter );
|
|
|
|
/* Events */
|
|
|
|
/**
|
|
* @event seen
|
|
* @param {boolean} [seen] Notification is seen
|
|
*
|
|
* Seen status of the notification has changed
|
|
*/
|
|
|
|
/**
|
|
* @event read
|
|
* @param {boolean} [read] Notification is read
|
|
*
|
|
* Read status of the notification has changed
|
|
*/
|
|
|
|
/* Methods */
|
|
|
|
/**
|
|
* Get NotificationItem id
|
|
* @return {string} NotificationItem Id
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.getId = function () {
|
|
return this.id;
|
|
};
|
|
|
|
/**
|
|
* Get NotificationItem content
|
|
* @return {jQuery|string} NotificationItem content
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.getContent = function () {
|
|
return this.content;
|
|
};
|
|
|
|
/**
|
|
* Get NotificationItem category
|
|
* @return {string} NotificationItem category
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.getCategory = function () {
|
|
return this.category;
|
|
};
|
|
|
|
/**
|
|
* Check whether this notification item is read
|
|
* @return {boolean} Notification item is read
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.isRead = function () {
|
|
return this.read;
|
|
};
|
|
|
|
/**
|
|
* Check whether this notification item is seen
|
|
* @return {boolean} Notification item is seen
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.isSeen = function () {
|
|
return this.seen;
|
|
};
|
|
|
|
/**
|
|
* Toggle the read state of the widget
|
|
*
|
|
* @param {boolean} [read] The current read state. If not given, the state will
|
|
* become the opposite of its current state.
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.toggleRead = function ( read ) {
|
|
read = read !== undefined ? read : !this.read;
|
|
if ( this.read !== read ) {
|
|
this.read = read;
|
|
this.emit( 'read', this.read );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Toggle the seen state of the widget
|
|
*
|
|
* @param {boolean} [seen] The current seen state. If not given, the state will
|
|
* become the opposite of its current state.
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.toggleSeen = function ( seen ) {
|
|
seen = seen !== undefined ? seen : !this.seen;
|
|
if ( this.seen !== seen ) {
|
|
this.seen = seen;
|
|
this.emit( 'seen', this.seen );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Set the notification timestamp
|
|
*
|
|
* @param {number} timestamp Notification timestamp in Mediawiki timestamp format
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.setTimestamp = function ( timestamp ) {
|
|
this.timestamp = Number( timestamp );
|
|
};
|
|
|
|
/**
|
|
* Get the notification timestamp
|
|
*
|
|
* @return {number} Notification timestamp in Mediawiki timestamp format
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.getTimestamp = function () {
|
|
return this.timestamp;
|
|
};
|
|
|
|
/**
|
|
* Set the notification link
|
|
*
|
|
* @param {string} link Notification url
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.setPrimaryUrl = function ( link ) {
|
|
this.primaryUrl = link;
|
|
};
|
|
|
|
/**
|
|
* Get the notification link
|
|
*
|
|
* @return {string} Notification url
|
|
*/
|
|
mw.echo.dm.NotificationItem.prototype.getPrimaryUrl = function () {
|
|
return this.primaryUrl;
|
|
};
|
|
|
|
}( mediaWiki, jQuery ) );
|