mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-28 09:40:41 +00:00
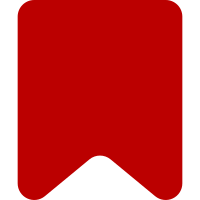
1) send apns topic when present in subscription metadata 2) check if subscription metadata is a valid JSON string 3) make epp_id column at echo_push_provider table auto_increment, otherwise it will fail when trying to add a second row in the table Bug: T259394 Change-Id: I785435e9f2d4ba9c14977d431d271f0fa2d0c795
136 lines
3.3 KiB
PHP
136 lines
3.3 KiB
PHP
<?php
|
|
|
|
namespace EchoPush\Api;
|
|
|
|
use ApiBase;
|
|
use ApiMain;
|
|
use EchoPush\SubscriptionManager;
|
|
use EchoServices;
|
|
use OverflowException;
|
|
use Wikimedia\ParamValidator\ParamValidator;
|
|
|
|
class ApiEchoPushSubscriptionsCreate extends ApiBase {
|
|
|
|
/**
|
|
* Supported push notification providers:
|
|
* (1) fcm: Firebase Cloud Messaging
|
|
* (2) apns: Apple Push Notification Service
|
|
*/
|
|
private const PROVIDERS = [ 'fcm', 'apns' ];
|
|
|
|
/** @var ApiBase */
|
|
private $parent;
|
|
|
|
/** @var SubscriptionManager */
|
|
private $subscriptionManager;
|
|
|
|
/**
|
|
* Static entry point for initializing the module
|
|
* @param ApiBase $parent Parent module
|
|
* @param string $name Module name
|
|
* @return ApiEchoPushSubscriptionsCreate
|
|
*/
|
|
public static function factory( ApiBase $parent, string $name ):
|
|
ApiEchoPushSubscriptionsCreate {
|
|
$subscriptionManger = EchoServices::getInstance()->getPushSubscriptionManager();
|
|
$module = new self( $parent->getMain(), $name, $subscriptionManger );
|
|
$module->parent = $parent;
|
|
return $module;
|
|
}
|
|
|
|
/**
|
|
* @param ApiMain $mainModule
|
|
* @param string $moduleName
|
|
* @param SubscriptionManager $subscriptionManager
|
|
*/
|
|
public function __construct(
|
|
ApiMain $mainModule,
|
|
string $moduleName,
|
|
SubscriptionManager $subscriptionManager
|
|
) {
|
|
parent::__construct( $mainModule, $moduleName );
|
|
$this->subscriptionManager = $subscriptionManager;
|
|
}
|
|
|
|
/**
|
|
* Entry point for executing the module.
|
|
* @inheritDoc
|
|
*/
|
|
public function execute(): void {
|
|
$provider = $this->getParameter( 'provider' );
|
|
$token = $this->getParameter( 'providertoken' );
|
|
$topic = null;
|
|
// check if metadata is a JSON string correctly encoded
|
|
if ( $provider === 'apns' ) {
|
|
$topic = $this->getParameter( 'topic' );
|
|
if ( !$topic ) {
|
|
$this->dieWithError( 'apierror-echo-push-topic-required' );
|
|
}
|
|
}
|
|
try {
|
|
$success = $this->subscriptionManager->create( $this->getUser(), $provider, $token, $topic );
|
|
} catch ( OverflowException $e ) {
|
|
$maxSubscriptionsPerUser = $this->subscriptionManager->getMaxSubscriptionsPerUser();
|
|
$this->dieWithError( [ 'apierror-echo-push-too-many-subscriptions',
|
|
$maxSubscriptionsPerUser ] );
|
|
}
|
|
|
|
if ( !$success ) {
|
|
$this->dieWithError( 'apierror-echo-push-token-exists' );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Get the parent module.
|
|
* @return ApiBase
|
|
*/
|
|
public function getParent(): ApiBase {
|
|
return $this->parent;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
protected function getAllowedParams(): array {
|
|
return [
|
|
'provider' => [
|
|
ParamValidator::PARAM_TYPE => self::PROVIDERS,
|
|
ParamValidator::PARAM_REQUIRED => true,
|
|
],
|
|
'providertoken' => [
|
|
ParamValidator::PARAM_TYPE => 'string',
|
|
ParamValidator::PARAM_REQUIRED => true,
|
|
],
|
|
'topic' => [
|
|
ParamValidator::PARAM_TYPE => 'string',
|
|
ParamValidator::PARAM_REQUIRED => false,
|
|
],
|
|
];
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
protected function getExamplesMessages(): array {
|
|
return [
|
|
"action=echopushsubscriptions&command=create&provider=fcm&providertoken=ABC123" =>
|
|
"apihelp-echopushsubscriptions+create-example"
|
|
];
|
|
}
|
|
|
|
// The parent module already enforces these but they make documentation nicer.
|
|
|
|
/** @inheritDoc */
|
|
public function isWriteMode(): bool {
|
|
return true;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function mustBePosted(): bool {
|
|
return true;
|
|
}
|
|
|
|
/** @inheritDoc */
|
|
public function isInternal(): bool {
|
|
// experimental!
|
|
return true;
|
|
}
|
|
|
|
}
|