mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-15 03:35:01 +00:00
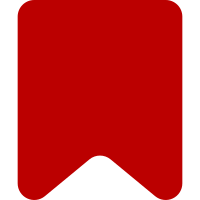
Update NPM packages: webdriverio, wdio-mediawiki. Replace NPM packages: - wdio-mocha-framework with @wdio/mocha-framework. - wdio-spec-reporter with @wdio/spec-reporter. New NPM packages: @wdio/cli, @wdio/local-runner, @wdio/sync. Replace: - `browser.element` with `$`. - `chromeOptions` with `'goog:chromeOptions'`. - `password` with `mwPwd`. - `username` with `mwUser`. - `waitForVisible()` with `waitForDisplayed()`. Bug: T250610 Change-Id: If7a5ee9588f082be18f27ee45a051ae956c688f5
63 lines
1.6 KiB
JavaScript
63 lines
1.6 KiB
JavaScript
'use strict';
|
||
|
||
const assert = require( 'assert' ),
|
||
EchoPage = require( '../pageobjects/echo.page' ),
|
||
UserLoginPage = require( 'wdio-mediawiki/LoginPage' ),
|
||
Util = require( 'wdio-mediawiki/Util' ),
|
||
Api = require( 'wdio-mediawiki/Api' );
|
||
|
||
describe( 'Echo', function () {
|
||
let bot;
|
||
|
||
before( async () => {
|
||
bot = await Api.bot();
|
||
} );
|
||
|
||
it( 'alerts and notices are visible after logging in @daily', function () {
|
||
|
||
UserLoginPage.login( browser.config.mwUser, browser.config.mwPwd );
|
||
|
||
assert( EchoPage.alerts.isExisting() );
|
||
assert( EchoPage.notices.isExisting() );
|
||
|
||
} );
|
||
|
||
it( 'flyout for alert appears when clicked @daily', function () {
|
||
|
||
UserLoginPage.login( browser.config.mwUser, browser.config.mwPwd );
|
||
EchoPage.alerts.click();
|
||
EchoPage.alertsFlyout.waitForDisplayed();
|
||
|
||
assert( EchoPage.alertsFlyout.isExisting() );
|
||
|
||
} );
|
||
|
||
it( 'flyout for notices appears when clicked @daily', function () {
|
||
|
||
UserLoginPage.login( browser.config.mwUser, browser.config.mwPwd );
|
||
EchoPage.notices.click();
|
||
EchoPage.noticesFlyout.waitForDisplayed();
|
||
|
||
assert( EchoPage.noticesFlyout.isExisting() );
|
||
|
||
} );
|
||
|
||
it( 'checks for welcome message after signup', function () {
|
||
|
||
const username = Util.getTestString( 'NewUser-' );
|
||
const password = Util.getTestString();
|
||
browser.call( async () => {
|
||
await Api.createAccount( bot, username, password );
|
||
} );
|
||
UserLoginPage.login( username, password );
|
||
|
||
EchoPage.notices.click();
|
||
|
||
EchoPage.alertMessage.waitForDisplayed();
|
||
const regexp = /Welcome to .*, .*! We're glad you're here./;
|
||
assert( regexp.test( EchoPage.alertMessage.getText() ) );
|
||
|
||
} );
|
||
|
||
} );
|