mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
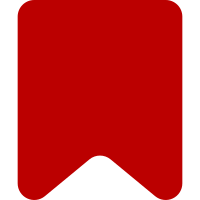
Class Revision is deprecated and in this patch, replaces usage with appropriate classes; RevisionRecord, RevisionStore, etc. Bug: T221163 Change-Id: Icfc85167a636bef95daab236ab80113c1a3cf41b
46 lines
906 B
PHP
46 lines
906 B
PHP
<?php
|
|
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
/**
|
|
* Cache class that maps revision id to RevisionStore object
|
|
*/
|
|
class EchoRevisionLocalCache extends EchoLocalCache {
|
|
|
|
/**
|
|
* @var EchoRevisionLocalCache
|
|
*/
|
|
private static $instance;
|
|
|
|
/**
|
|
* @return EchoRevisionLocalCache
|
|
*/
|
|
public static function create() {
|
|
if ( !self::$instance ) {
|
|
self::$instance = new EchoRevisionLocalCache();
|
|
}
|
|
|
|
return self::$instance;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
protected function resolve( array $lookups ) {
|
|
$store = MediaWikiServices::getInstance()->getRevisionStore();
|
|
$dbr = wfGetDB( DB_REPLICA );
|
|
$revQuery = $store->getQueryInfo( [ 'page', 'user' ] );
|
|
$res = $dbr->select(
|
|
$revQuery['tables'],
|
|
$revQuery['fields'],
|
|
[ 'rev_id' => $lookups ],
|
|
__METHOD__,
|
|
[],
|
|
$revQuery['joins']
|
|
);
|
|
foreach ( $res as $row ) {
|
|
yield $row->rev_id => $store->newRevisionFromRow( $row );
|
|
}
|
|
}
|
|
}
|