mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
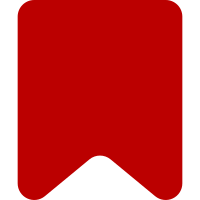
isRegistered is part of the slick UserIdentity interface, i.e. it's the more "canonical" form. This change makes it a bit easier to move away from using the huge (4000+ LOC) User class everywhere, in favor of the UserIdentity interface, where possible. This patch is meant as a small step towards this goal. I tried to replace some usages of User type hints already, but prefer to go in small, incremental steps. Change-Id: I039c7a18672dfb6ea9507752bce9ea754babd690
109 lines
2.8 KiB
PHP
109 lines
2.8 KiB
PHP
<?php
|
|
|
|
class ApiEchoArticleReminder extends ApiBase {
|
|
|
|
public function execute() {
|
|
$this->getMain()->setCacheMode( 'private' );
|
|
$user = $this->getUser();
|
|
if ( !$user->isRegistered() ) {
|
|
$this->dieWithError( 'apierror-mustbeloggedin-generic', 'login-required' );
|
|
}
|
|
|
|
$params = $this->extractRequestParams();
|
|
$result = [];
|
|
$userTimestamp = new MWTimestamp( $params['timestamp'] );
|
|
$nowTimestamp = new MWTimestamp();
|
|
// We need $params['timestamp'] to be a future timestamp:
|
|
// $userTimestamp < $nowTimestamp = invert 0
|
|
// $userTimestamp > $nowTimestamp = invert 1
|
|
if ( $userTimestamp->diff( $nowTimestamp )->invert === 0 ) {
|
|
$this->dieWithError( [ 'apierror-badparameter', 'timestamp' ], 'timestamp-not-in-future', null, 400 );
|
|
}
|
|
|
|
$eventCreation = EchoEvent::create( [
|
|
'type' => 'article-reminder',
|
|
'agent' => $user,
|
|
'title' => $this->getTitleFromTitleOrPageId( $params ),
|
|
'extra' => [
|
|
'comment' => $params['comment'],
|
|
],
|
|
] );
|
|
|
|
if ( !$eventCreation ) {
|
|
$this->dieWithError( 'apierror-echo-event-creation-failed', null, null, 500 );
|
|
}
|
|
|
|
/* Temp - removing the delay just for now:
|
|
$job = new JobSpecification(
|
|
'articleReminder',
|
|
[
|
|
'userId' => $user->getId(),
|
|
'timestamp' => $params['timestamp'],
|
|
'comment' => $params['comment'],
|
|
],
|
|
[ 'removeDuplicates' => true ],
|
|
Title::newFromID( $params['pageid'] )
|
|
);
|
|
JobQueueGroup::singleton()->push( $job );*/
|
|
$result += [
|
|
'result' => 'success'
|
|
];
|
|
$this->getResult()->addValue( 'query', $this->getModuleName(), $result );
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return [
|
|
'pageid' => [
|
|
ApiBase::PARAM_TYPE => 'integer',
|
|
],
|
|
'title' => [
|
|
ApiBase::PARAM_TYPE => 'string',
|
|
],
|
|
'comment' => [
|
|
ApiBase::PARAM_TYPE => 'string',
|
|
],
|
|
'timestamp' => [
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
ApiBase::PARAM_TYPE => 'timestamp',
|
|
],
|
|
'token' => [
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
],
|
|
];
|
|
}
|
|
|
|
public function needsToken() {
|
|
return 'csrf';
|
|
}
|
|
|
|
public function mustBePosted() {
|
|
return true;
|
|
}
|
|
|
|
public function isWriteMode() {
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
* @return string[]
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
$todayDate = new DateTime();
|
|
$oneDay = new DateInterval( 'P1D' );
|
|
$tomorrowDate = $todayDate->add( $oneDay );
|
|
$tomorrowDateTimestamp = new MWTimestamp( $tomorrowDate );
|
|
$tomorrowTimestampStr = $tomorrowDateTimestamp->getTimestamp( TS_ISO_8601 );
|
|
return [
|
|
"action=echoarticlereminder&pageid=1×tamp=$tomorrowTimestampStr&comment=example"
|
|
=> 'apihelp-echoarticlereminder-example-1',
|
|
"action=echoarticlereminder&title=Main_Page×tamp=$tomorrowTimestampStr"
|
|
=> 'apihelp-echoarticlereminder-example-2',
|
|
];
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Special:MyLanguage/Echo_(Notifications)/API';
|
|
}
|
|
}
|