mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-24 18:59:40 +00:00
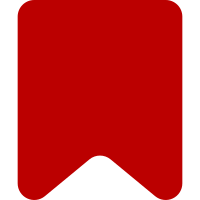
* In some languages like persian, the number 0 is represented as '.', we can't compare '.' with either 0 or '0' to detect the no-notification status of the badge * The markread API doesn't respect uselang param, it would return 0 instead of . in a url with uselang=fa Note: we need to provide raw and formatted count in the API since client side javascript doesn't provide fancy function like $wgLang->formatNum() bug: 54575 Change-Id: I0a49828253ec346ed27c5b9a976f8bdff4e1fa90
102 lines
2.3 KiB
PHP
102 lines
2.3 KiB
PHP
<?php
|
|
|
|
class ApiEchoMarkRead extends ApiBase {
|
|
|
|
public function __construct( $query, $moduleName ) {
|
|
parent::__construct( $query, $moduleName );
|
|
}
|
|
|
|
public function execute() {
|
|
// To avoid API warning, register the parameter used to bust browser cache
|
|
$this->getMain()->getVal( '_' );
|
|
|
|
$user = $this->getUser();
|
|
if ( $user->isAnon() ) {
|
|
$this->dieUsage( 'Login is required', 'login-required' );
|
|
}
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( $user );
|
|
|
|
$params = $this->extractRequestParams();
|
|
|
|
// There is no need to trigger markRead if all notifications are read
|
|
if ( $notifUser->getNotificationCount() > 0 ) {
|
|
if ( count( $params['list'] ) ) {
|
|
// Make sure there is a limit to the update
|
|
$notifUser->markRead( array_slice( $params['list'], 0, ApiBase::LIMIT_SML2 ) );
|
|
} elseif ( $params['all'] ) {
|
|
$notifUser->markAllRead();
|
|
}
|
|
}
|
|
|
|
$rawCount = $notifUser->getNotificationCount();
|
|
|
|
$result = array(
|
|
'result' => 'success',
|
|
'rawcount' => $rawCount,
|
|
'count' => EchoNotificationController::formatNotificationCount( $rawCount ),
|
|
);
|
|
$this->getResult()->addValue( 'query', $this->getModuleName(), $result );
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return array(
|
|
'list' => array(
|
|
ApiBase::PARAM_ISMULTI => true,
|
|
),
|
|
'all' => array(
|
|
ApiBase::PARAM_REQUIRED => false,
|
|
ApiBase::PARAM_TYPE => 'boolean'
|
|
),
|
|
'token' => array(
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
),
|
|
'uselang' => null
|
|
);
|
|
}
|
|
|
|
public function getParamDescription() {
|
|
return array(
|
|
'list' => 'A list of notification IDs to mark as read',
|
|
'all' => "If set to true, marks all of a user's notifications as read",
|
|
'token' => 'edit token',
|
|
'uselang' => 'the desired language to format the output'
|
|
);
|
|
}
|
|
|
|
public function needsToken() {
|
|
return true;
|
|
}
|
|
|
|
public function getTokenSalt() {
|
|
return '';
|
|
}
|
|
|
|
public function mustBePosted() {
|
|
return true;
|
|
}
|
|
|
|
public function isWriteMode() {
|
|
return true;
|
|
}
|
|
|
|
public function getDescription() {
|
|
return 'Mark notifications as read for the current user';
|
|
}
|
|
|
|
public function getExamples() {
|
|
return array(
|
|
'api.php?action=echomarkread&list=8',
|
|
'api.php?action=echomarkread&all=true'
|
|
);
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Echo_(notifications)/API';
|
|
}
|
|
|
|
public function getVersion() {
|
|
return __CLASS__ . '-0.1';
|
|
}
|
|
}
|