mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-12-04 04:09:00 +00:00
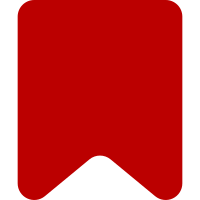
Also adds an API module for muting and unmuting pages (and users). Bug: T46787 Bug: T115264 Change-Id: Icf4e4bfa9fd7fa27b4c40892e3d5ce000eb22d5a
75 lines
1.7 KiB
PHP
75 lines
1.7 KiB
PHP
<?php
|
|
|
|
use Wikimedia\TestingAccessWrapper;
|
|
|
|
/**
|
|
* @coversDefaultClass EchoNotificationController
|
|
*/
|
|
class NotificationControllerUnitTest extends MediaWikiUnitTestCase {
|
|
|
|
/**
|
|
* @dataProvider PageLinkedTitleMutedByUserDataProvider
|
|
* @covers ::isPageLinkedTitleMutedByUser
|
|
* @param Title $title
|
|
* @param User $user
|
|
* @param bool $expected
|
|
*/
|
|
public function testIsPageLinkedTitleMutedByUser(
|
|
Title $title, User $user, bool $expected ): void {
|
|
$wrapper = TestingAccessWrapper::newFromClass( EchoNotificationController::class );
|
|
$wrapper->mutedPageLinkedTitlesCache = $this->getMapCacheLruMock();
|
|
$this->assertSame(
|
|
$expected,
|
|
$wrapper->isPageLinkedTitleMutedByUser( $title, $user )
|
|
);
|
|
}
|
|
|
|
public function PageLinkedTitleMutedByUserDataProvider() :array {
|
|
return [
|
|
[
|
|
$this->getMockTitle( 123 ),
|
|
$this->getMockUser( [] ),
|
|
false
|
|
],
|
|
[
|
|
$this->getMockTitle( 123 ),
|
|
$this->getMockUser( [ 123, 456, 789 ] ),
|
|
true
|
|
],
|
|
[
|
|
$this->getMockTitle( 456 ),
|
|
$this->getMockUser( [ 489 ] ),
|
|
false
|
|
]
|
|
|
|
];
|
|
}
|
|
|
|
private function getMockTitle( int $articleID ) {
|
|
$title = $this->getMockBuilder( Title::class )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$title->method( 'getArticleID' )
|
|
->willReturn( $articleID );
|
|
return $title;
|
|
}
|
|
|
|
private function getMockUser( $mutedTitlePreferences = [] ) {
|
|
$user = $this->getMockBuilder( User::class )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
$user->method( 'getId' )
|
|
->willReturn( 456 );
|
|
$user->method( 'getOption' )
|
|
->willReturn( implode( "\n", $mutedTitlePreferences ) );
|
|
return $user;
|
|
}
|
|
|
|
private function getMapCacheLruMock() {
|
|
return $this->getMockBuilder( MapCacheLRU::class )
|
|
->disableOriginalConstructor()
|
|
->getMock();
|
|
}
|
|
|
|
}
|