mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 03:09:37 +00:00
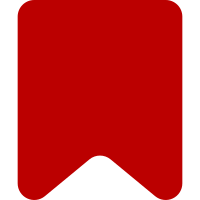
Other tests (possibly from other enabled extensions) could also, by chance, create Echo notifications for the user MWEchoThankYouEditTests uses. In order to those tests be reliable, they should make sure there is no notification data in the database prior to running tests. Bug: T161087 Change-Id: I870a50b1f831795731235fa8ec97477b3e470b50
69 lines
2.1 KiB
PHP
69 lines
2.1 KiB
PHP
<?php
|
|
|
|
/**
|
|
* @group Echo
|
|
*/
|
|
class MWEchoThankYouEditTest extends MediaWikiTestCase {
|
|
|
|
protected function setUp() {
|
|
parent::setUp();
|
|
$this->tablesUsed[] = 'echo_event';
|
|
$this->tablesUsed[] = 'echo_notification';
|
|
}
|
|
|
|
private function deleteEchoData() {
|
|
$db = MWEchoDbFactory::newFromDefault()->getEchoDb( DB_MASTER );
|
|
$db->delete( 'echo_event', '*', __METHOD__ );
|
|
$db->delete( 'echo_notification', '*', __METHOD__ );
|
|
}
|
|
|
|
public function testFirstEdit() {
|
|
// setup
|
|
$this->deleteEchoData();
|
|
$user = $this->getMutableTestUser()->getUser();
|
|
$title = Title::newFromText( 'Help:MWEchoThankYouEditTest_testFirstEdit' );
|
|
|
|
// action
|
|
$this->edit( $title, $user, 'this is my first edit' );
|
|
|
|
// assertions
|
|
$notificationMapper = new EchoNotificationMapper();
|
|
$notifications = $notificationMapper->fetchByUser( $user, 10, null, [ 'thank-you-edit' ] );
|
|
$this->assertCount( 1, $notifications );
|
|
|
|
/** @var EchoNotification $notification */
|
|
$notification = reset( $notifications );
|
|
$this->assertEquals( 1, $notification->getEvent()->getExtraParam( 'editCount', 'not found' ) );
|
|
}
|
|
|
|
public function testTenthEdit() {
|
|
// setup
|
|
$this->deleteEchoData();
|
|
$user = $this->getMutableTestUser()->getUser();
|
|
$title = Title::newFromText( 'Help:MWEchoThankYouEditTest_testTenthEdit' );
|
|
|
|
// action
|
|
// we could fast-forward the edit-count to speed things up
|
|
// but this is the only way to make sure duplicate notifications
|
|
// are not generated
|
|
for ( $i = 0; $i < 12; $i++ ) {
|
|
$this->edit( $title, $user, "this is edit #$i" );
|
|
}
|
|
|
|
// assertions
|
|
$notificationMapper = new EchoNotificationMapper();
|
|
$notifications = $notificationMapper->fetchByUser( $user, 10, null, [ 'thank-you-edit' ] );
|
|
$this->assertCount( 2, $notifications );
|
|
|
|
/** @var EchoNotification $notification */
|
|
$notification = reset( $notifications );
|
|
$this->assertEquals( 10, $notification->getEvent()->getExtraParam( 'editCount', 'not found' ) );
|
|
}
|
|
|
|
private function edit( Title $title, User $user, $text ) {
|
|
$page = WikiPage::factory( $title );
|
|
$content = ContentHandler::makeContent( $text, $title );
|
|
$page->doEditContent( $content, 'test', 0, false, $user );
|
|
}
|
|
}
|