mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 11:17:49 +00:00
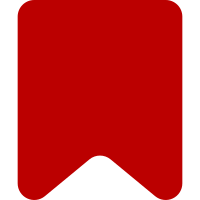
This needs some more manual testing and adding unit testing Change-Id: Iadfe3cf7927d5318f89ba17f067000f9399060af
73 lines
2 KiB
PHP
73 lines
2 KiB
PHP
<?php
|
|
|
|
class MWEchoNotifUserTest extends MediaWikiTestCase {
|
|
|
|
protected function setUp() {
|
|
parent::setUp();
|
|
$this->setMwGlobals( 'wgMemc', new HashBagOStuff() );
|
|
}
|
|
|
|
public function testNewFromUser() {
|
|
$exception = false;
|
|
try {
|
|
MWEchoNotifUser::newFromUser( User::newFromId( 0 ) );
|
|
} catch ( Exception $e ) {
|
|
$exception = true;
|
|
$this->assertEquals( "User must be logged in to view notification!",
|
|
$e->getMessage() );
|
|
}
|
|
$this->assertTrue( $exception, "Got exception" );
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( User::newFromId( 2 ) );
|
|
$this->assertInstanceOf( 'MWEchoNotifUser', $notifUser );
|
|
}
|
|
|
|
public function testFlagCacheWithNewTalkNotification() {
|
|
global $wgMemc;
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( User::newFromId( 2 ) );
|
|
|
|
$notifUser->flagCacheWithNewTalkNotification();
|
|
$this->assertEquals( '1', $wgMemc->get( $notifUser->getTalkNotificationCacheKey() ) );
|
|
}
|
|
|
|
public function testFlagCacheWithNoTalkNotification() {
|
|
global $wgMemc;
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( User::newFromId( 2 ) );
|
|
|
|
$notifUser->flagCacheWithNoTalkNotification();
|
|
$this->assertEquals( '0', $wgMemc->get( $notifUser->getTalkNotificationCacheKey() ) );
|
|
}
|
|
|
|
public function testNotifCountHasReachedMax() {
|
|
global $wgEchoMaxNotificationCount;
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( User::newFromId( 2 ) );
|
|
|
|
if ( $notifUser->getNotificationCount() > $wgEchoMaxNotificationCount ) {
|
|
$this->assertTrue( $notifUser->notifCountHasReachedMax() );
|
|
} else {
|
|
$this->assertFalse( $notifUser->notifCountHasReachedMax() );
|
|
}
|
|
}
|
|
|
|
public function testClearTalkNotification() {
|
|
global $wgMemc;
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( User::newFromId( 2 ) );
|
|
|
|
$notifUser->flagCacheWithNewTalkNotification();
|
|
|
|
$hasMax = $notifUser->notifCountHasReachedMax();
|
|
|
|
$notifUser->clearTalkNotification();
|
|
if ( $hasMax ) {
|
|
$this->assertEquals( '1', $wgMemc->get( $notifUser->getTalkNotificationCacheKey() ) );
|
|
} else {
|
|
$this->assertEquals( '0', $wgMemc->get( $notifUser->getTalkNotificationCacheKey() ) );
|
|
}
|
|
}
|
|
|
|
}
|