mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-25 00:05:29 +00:00
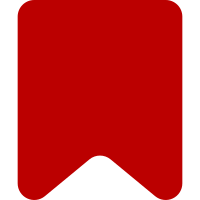
Some complexity is now gone. We didn't currently have a good justification for a the APIHandler factory: the apiHandler caller would have to specify (variable `foreign`) what kind of handler it would like to initiate anyway, so it might as well just inject the object (which makes the code easier to follow, decreases bugs risk because there are less code paths) This also gives the caller more control of the API handlers: registerForeignSources will now be able to do more. Now it can e.g. create 1 object that is shared for multiple wikis (to do lookups for multiple wikis at once) Also renamed addApiHandler to setApiHandler (it just sets the value it needs without checking if it already existed anyway) Change-Id: Ie1814c5bf1a1f0e5607033beb506df67f3585b24
149 lines
5.5 KiB
JavaScript
149 lines
5.5 KiB
JavaScript
( function ( mw ) {
|
|
/**
|
|
* A class defining Echo API instructions and network operations
|
|
*
|
|
* @constructor
|
|
*/
|
|
mw.echo.api.EchoApi = function MwEchoApiEchoApi() {
|
|
this.network = new mw.echo.api.NetworkHandler();
|
|
|
|
this.fetchingPromise = null;
|
|
};
|
|
|
|
OO.initClass( mw.echo.api.EchoApi );
|
|
|
|
/**
|
|
* Register a set of sources.
|
|
*
|
|
* @param {Object} sources Object mapping source names to config objects
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.registerForeignSources = function ( sources ) {
|
|
var s;
|
|
for ( s in sources ) {
|
|
this.network.setApiHandler( s, new mw.echo.api.ForeignAPIHandler( sources[ s ] ) );
|
|
}
|
|
};
|
|
|
|
/**
|
|
* Fetch notifications from the server based on type
|
|
*
|
|
* @param {string} type Notification type to fetch: 'alert', 'message', or 'all'
|
|
* @param {string|string[]} [sources] The source from which to fetch the notifications.
|
|
* If not given, the local notifications will be fetched.
|
|
* @param {boolean} [isForced] Force a refresh on the fetch notifications promise
|
|
* @return {jQuery.Promise} Promise that is resolved with all notifications for the
|
|
* requested types.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.fetchNotifications = function ( type, sources, isForced ) {
|
|
sources = Array.isArray( sources ) ?
|
|
sources :
|
|
sources ?
|
|
[ sources ] :
|
|
null;
|
|
|
|
return this.network.getApiHandler( 'local' ).fetchNotifications( type, sources, isForced )
|
|
.then( function ( result ) {
|
|
return OO.getProp( result.query, 'notifications' );
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* Fetch notifications from several sources
|
|
*
|
|
* @param {string[]} sourceArray An array of sources to fetch from the group
|
|
* @param {string} type Notification type
|
|
* @return {jQuery.Promise} A promise that resolves with an array of promises for
|
|
* fetchNotifications per each given source in the source array.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.fetchNotificationGroups = function ( sourceArray, type ) {
|
|
return this.network.getApiHandler( 'local' ).fetchNotifications( type, sourceArray )
|
|
.then( function ( result ) {
|
|
return OO.getProp( result, 'query', 'notifications', 'list' );
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* Mark items as read in the API.
|
|
*
|
|
* @param {string[]} itemIds An array of item IDs to mark as read
|
|
* @param {string} source The source that these items belong to
|
|
* @param {boolean} [isRead] The read state of the item; true for marking the
|
|
* item as read, false for marking the item as unread
|
|
* @return {jQuery.Promise} A promise that is resolved when the operation
|
|
* is complete, with the number of unread notifications still remaining
|
|
* for that type in the given source
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.markItemsRead = function ( itemIds, source, isRead ) {
|
|
return this.network.getApiHandler( source ).markItemsRead( itemIds, isRead );
|
|
};
|
|
|
|
/**
|
|
* Mark all notifications for a given type as read in the given source.
|
|
*
|
|
* @param {string} source Symbolic name of notifications source
|
|
* @param {string} type Notifications type
|
|
* @return {jQuery.Promise} A promise that is resolved when the operation
|
|
* is complete, with the number of unread notifications still remaining
|
|
* for that type in the given source
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.markAllRead = function ( source, type ) {
|
|
// FIXME: This specific method sends an operation
|
|
// to the API that marks all notifications of the given type as read regardless
|
|
// of whether they were actually seen by the user.
|
|
// We should consider removing the use of this method and, instead,
|
|
// using strictly the 'markItemsRead' by giving the API only the
|
|
// notifications that are available to the user.
|
|
return this.network.getApiHandler( source ).markAllRead( type );
|
|
};
|
|
|
|
/**
|
|
* Fetch the number of unread notifications for the given type in the given
|
|
* source.
|
|
*
|
|
* @param {string} source Notifications source
|
|
* @param {string} type Notification type
|
|
* @return {jQuery.Promise} A promise that is resolved with the number of
|
|
* unread notifications for the given type and source.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.fetchUnreadCount = function ( source, type ) {
|
|
return this.network.getApiHandler( source ).fetchUnreadCount( type );
|
|
};
|
|
|
|
/**
|
|
* Update the seenTime property for the given type and source.
|
|
*
|
|
* @param {string} source Notification source
|
|
* @param {string} type Notification type
|
|
* @return {jQuery.Promise} A promise that is resolved when the operation is complete.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.updateSeenTime = function ( source, type ) {
|
|
return this.network.getApiHandler( source ).updateSeenTime( type );
|
|
};
|
|
|
|
/**
|
|
* Check whether the API promise for fetch notification is in an error
|
|
* state for the given source and notification type.
|
|
*
|
|
* @param {string} source Notification source.
|
|
* @param {string} type Notification type
|
|
* @return {boolean} The API response for fetching notification has
|
|
* resolved in an error state, or is rejected.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.isFetchingErrorState = function ( source, type ) {
|
|
return this.network.getApiHandler( source ).isFetchingErrorState( type, [ source ] );
|
|
};
|
|
|
|
/**
|
|
* Get the fetch notifications promise active for the current source and type.
|
|
*
|
|
* @param {string} source Notification source.
|
|
* @param {string} type Notification type
|
|
* @return {jQuery.Promise} Promise that is resolved when notifications are
|
|
* fetched from the API.
|
|
*/
|
|
mw.echo.api.EchoApi.prototype.getFetchNotificationPromise = function ( source, type ) {
|
|
return this.network.getApiHandler( source ).getFetchNotificationPromise( type );
|
|
};
|
|
|
|
} )( mediaWiki );
|