mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 03:09:37 +00:00
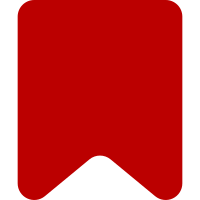
All files containing more than one PHP class were split into multiple files. extension.json was updated to match new class locations. Bug: T177809 Change-Id: I4e7d8f02164c3048c41c4c9fbe4be18a99e7abaa
55 lines
1.3 KiB
PHP
55 lines
1.3 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Implements EchoContainmentList interface for sourcing a list of items from a wiki
|
|
* page. Uses the pages latest revision ID as cache key.
|
|
*/
|
|
class EchoOnWikiList implements EchoContainmentList {
|
|
/**
|
|
* @var Title|null A title object representing the page to source the list from,
|
|
* or null if the page does not exist.
|
|
*/
|
|
protected $title;
|
|
|
|
/**
|
|
* @param int $titleNs An NS_* constant representing the mediawiki namespace of the page
|
|
* @param string $titleString String portion of the wiki page title
|
|
*/
|
|
public function __construct( $titleNs, $titleString ) {
|
|
$title = Title::newFromText( $titleString, $titleNs );
|
|
if ( $title !== null && $title->getArticleId() ) {
|
|
$this->title = $title;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getValues() {
|
|
if ( !$this->title ) {
|
|
return [];
|
|
}
|
|
|
|
$article = WikiPage::newFromID( $this->title->getArticleId() );
|
|
if ( $article === null || !$article->exists() ) {
|
|
return [];
|
|
}
|
|
$text = ContentHandler::getContentText( $article->getContent() );
|
|
if ( $text === null ) {
|
|
return [];
|
|
}
|
|
return array_filter( array_map( 'trim', explode( "\n", $text ) ) );
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getCacheKey() {
|
|
if ( !$this->title ) {
|
|
return '';
|
|
}
|
|
|
|
return $this->title->getLatestRevID();
|
|
}
|
|
}
|