mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-23 18:30:06 +00:00
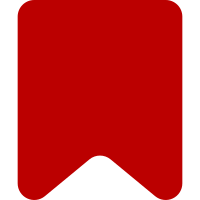
The change to use DeferredUpdates had a missing use. Change-Id: Ied72608c9b3aa597627aa5ea069c257f506c3c1b
42 lines
1.3 KiB
PHP
42 lines
1.3 KiB
PHP
<?php
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
/**
|
|
* This class represents the controller for moderating notifications
|
|
*/
|
|
class EchoModerationController {
|
|
|
|
/**
|
|
* Moderate or unmoderate events
|
|
*
|
|
* @param int[] $eventIds
|
|
* @param bool $moderate Whether to moderate or unmoderate the events
|
|
* @throws MWException
|
|
*/
|
|
public static function moderate( $eventIds, $moderate ) {
|
|
if ( !$eventIds ) {
|
|
return;
|
|
}
|
|
|
|
$eventMapper = new EchoEventMapper();
|
|
$notificationMapper = new EchoNotificationMapper();
|
|
|
|
$affectedUserIds = $notificationMapper->fetchUsersWithNotificationsForEvents( $eventIds );
|
|
$eventMapper->toggleDeleted( $eventIds, $moderate );
|
|
|
|
DeferredUpdates::addCallableUpdate( function () use ( $affectedUserIds ) {
|
|
// This update runs after the main transaction round commits.
|
|
// Wait for the event deletions to be propagated to replica DBs
|
|
$lbFactory = MediaWikiServices::getInstance()->getDBLoadBalancerFactory();
|
|
$lbFactory->waitForReplication( [ 'timeout' => 5 ] );
|
|
$lbFactory->flushReplicaSnapshots( 'EchoModerationController::moderate' );
|
|
// Recompute the notification count for the
|
|
// users whose notifications have been moderated.
|
|
foreach ( $affectedUserIds as $userId ) {
|
|
$user = User::newFromId( $userId );
|
|
MWEchoNotifUser::newFromUser( $user )->resetNotificationCount( DB_REPLICA );
|
|
}
|
|
} );
|
|
}
|
|
}
|