mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-24 02:39:33 +00:00
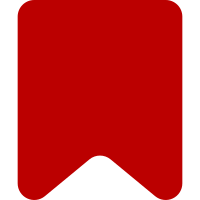
This involves: * Making this value no longer admin-configurable. * Changing getNotificationCountForOutput to return only a single value Since there is no + in the formatted value anymore, we can actually use the same value for both. This is a B/C break, but hopefully worth it to simplify the method call. For now, the excess parameter is just marked unused. It could be removed at some point if the translations are updated. This must be merged at the same time as: * Flow - Ibfa56b1af9e8c56b4c5f900e0d487bc09688b2a2 * MobileFrontend - Ibf784b279d56773a227ff261b75f2b26125bbb63 (well, MF can be merged first) * translatewiki - I2a4b6938aed49e4101deb6b9351c43656a863490 Also, change 1 to One/one, per Siebrand on the task. This can easily be dropped/undone if we don't want it. Also, remove reference to no-longer-existent notification-page-linked-bundle Bug: T127288 Change-Id: Iabeaae747f99980c0610d552f6b38f89d940b890
83 lines
2.5 KiB
PHP
83 lines
2.5 KiB
PHP
<?php
|
|
|
|
class EchoPageLinkedPresentationModel extends EchoEventPresentationModel {
|
|
|
|
public function getIconType() {
|
|
return 'linked';
|
|
}
|
|
|
|
/**
|
|
* The page containing the link may be a new page
|
|
* that is not yet replicated.
|
|
* This event won't be rendered unless/until
|
|
* both pages are available.
|
|
* @return bool
|
|
*/
|
|
public function canRender() {
|
|
$pageTo = $this->event->getTitle();
|
|
$pageFrom = $this->getPageFrom();
|
|
return (bool)$pageTo && (bool)$pageFrom;
|
|
}
|
|
|
|
public function getPrimaryLink() {
|
|
return array(
|
|
'url' => $this->getPageFrom()->getFullURL(),
|
|
'label' => $this->msg( 'notification-link-text-view-page' )->text(),
|
|
);
|
|
}
|
|
|
|
public function getSecondaryLinks() {
|
|
$whatLinksHereLink = array(
|
|
'url' => SpecialPage::getTitleFor( 'Whatlinkshere', $this->event->getTitle()->getPrefixedText() )->getFullURL(),
|
|
'label' => $this->msg( 'notification-link-text-what-links-here' )->text(),
|
|
'description' => '',
|
|
'icon' => 'linked',
|
|
'prioritized' => true
|
|
);
|
|
return array( $whatLinksHereLink );
|
|
}
|
|
|
|
protected function getHeaderMessageKey() {
|
|
if ( $this->getBundleCount( true, array( $this, 'getLinkedPageId' ) ) > 1 ) {
|
|
return "notification-bundle-header-{$this->type}";
|
|
}
|
|
return "notification-header-{$this->type}";
|
|
}
|
|
|
|
public function getHeaderMessage() {
|
|
$msg = parent::getHeaderMessage();
|
|
$msg->params( $this->getTruncatedTitleText( $this->event->getTitle(), true ) );
|
|
$msg->params( $this->getTruncatedTitleText( $this->getPageFrom(), true ) );
|
|
$count =
|
|
$this->getNotificationCountForOutput( false, array( $this, 'getLinkedPageId' ) );
|
|
|
|
// Repeat is B/C until unused parameter is removed from translations
|
|
$msg->numParams( $count, $count );
|
|
return $msg;
|
|
}
|
|
|
|
/**
|
|
* Get the page ID of the linked-from page for a given event.
|
|
* @param EchoEvent $event page-linked event
|
|
* @return int Page ID, or 0 if the page doesn't exist
|
|
*/
|
|
public function getLinkedPageId( EchoEvent $event ) {
|
|
$extra = $event->getExtra();
|
|
if ( isset( $extra['link-from-page-id'] ) ) {
|
|
return $extra['link-from-page-id'];
|
|
}
|
|
// Backwards compatiblity for events from before https://gerrit.wikimedia.org/r/#/c/63076
|
|
if ( isset( $extra['link-from-namespace'] ) && isset( $extra['link-from-title'] ) ) {
|
|
$title = Title::makeTitleSafe( $extra['link-from-namespace'], $extra['link-from-title'] );
|
|
if ( $title ) {
|
|
return $title->getArticleId();
|
|
}
|
|
}
|
|
return 0;
|
|
}
|
|
|
|
private function getPageFrom() {
|
|
return Title::newFromId( $this->getLinkedPageId( $this->event ) );
|
|
}
|
|
}
|