mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-24 02:39:33 +00:00
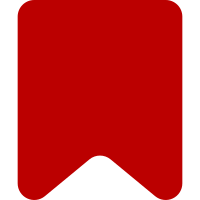
Formatters based on presentation models for individual event emails and digest (daily, weekly) plain text emails. Bug: T121067 Change-Id: I4eceaf521315adab7429a8a73ffca70ebcddab86
54 lines
1.3 KiB
PHP
54 lines
1.3 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Abstract class for formatters that process multiple events.
|
|
*
|
|
* The formatter does not maintain any state except for the
|
|
* arguments passed in the constructor (user and language)
|
|
*/
|
|
abstract class EchoEventDigestFormatter {
|
|
public function __construct( User $user, Language $language ) {
|
|
$this->user = $user;
|
|
$this->language = $language;
|
|
}
|
|
|
|
/**
|
|
* Equivalent to IContextSource::msg for the current
|
|
* language
|
|
*
|
|
* @return Message
|
|
*/
|
|
protected function msg( /* ,,, */ ) {
|
|
/**
|
|
* @var Message $msg
|
|
*/
|
|
$msg = call_user_func_array( 'wfMessage', func_get_args() );
|
|
$msg->inLanguage( $this->language );
|
|
|
|
return $msg;
|
|
}
|
|
|
|
/**
|
|
* @param EchoEvent[] $events
|
|
* @param string $distributionType 'web' or 'email'
|
|
* @return array|bool|string Output format depends on implementation, false if it cannot be formatted
|
|
*/
|
|
final public function format( array $events, $distributionType ) {
|
|
$models = array();
|
|
foreach ( $events as $event ) {
|
|
$model = EchoEventPresentationModel::factory( $event, $this->language, $this->user, $distributionType );
|
|
if ( $model->canRender() ) {
|
|
$models[] = $model;
|
|
}
|
|
}
|
|
|
|
return $this->formatModels( $models );
|
|
}
|
|
|
|
/**
|
|
* @param EchoEventPresentationModel[] $models
|
|
* @return string|array
|
|
*/
|
|
abstract protected function formatModels( array $models );
|
|
}
|