mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-15 03:35:01 +00:00
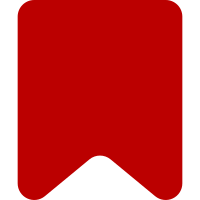
This is in preparation for dealing with cross-wiki notifications where we may need several types of operations to extract bundled notifications from local and external APIs. Also, renamed files: * mw.echo.dm.AbstractAPIHandler -> mw.echo.dm.APIHandler * mw.echo.dm.APIHandler -> mw.echo.dm.LocalAPIHandler * All API-related handler files moved to their own folder for better organization. Change-Id: Ib730c780ea52c93a6026c5d0b22012b6f39bb50d
117 lines
2.9 KiB
JavaScript
117 lines
2.9 KiB
JavaScript
( function ( mw, $ ) {
|
|
/**
|
|
* Notification API handler
|
|
*
|
|
* @class
|
|
* @extends mw.echo.dm.APIHandler
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Configuration object
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler = function MwEchoDmLocalAPIHandler( config ) {
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
mw.echo.dm.LocalAPIHandler.parent.call( this, config );
|
|
|
|
this.api = new mw.Api( { ajax: { cache: false } } );
|
|
};
|
|
|
|
/* Setup */
|
|
|
|
OO.inheritClass( mw.echo.dm.LocalAPIHandler, mw.echo.dm.APIHandler );
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler.prototype.fetchNotifications = function ( apiPromise ) {
|
|
var helper = this,
|
|
params = $.extend( { notsections: this.type }, this.getBaseParams() );
|
|
|
|
if ( !this.fetchNotificationsPromise || this.isFetchingErrorState() ) {
|
|
this.apiErrorState = false;
|
|
this.fetchNotificationsPromise = ( apiPromise || this.api.get( params ) )
|
|
.fail( function () {
|
|
// Mark API error state
|
|
helper.apiErrorState = true;
|
|
} )
|
|
.always( function () {
|
|
helper.fetchNotificationsPromise = null;
|
|
} );
|
|
}
|
|
|
|
return this.fetchNotificationsPromise;
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler.prototype.updateSeenTime = function ( type ) {
|
|
type = type || this.type;
|
|
|
|
return this.api.postWithToken( 'edit', {
|
|
action: 'echomarkseen',
|
|
// TODO: The API should also work with piped types like
|
|
// getting notification lists
|
|
type: $.isArray( type ) ? 'all' : type
|
|
} )
|
|
.then( function ( data ) {
|
|
return data.query.echomarkseen.timestamp;
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler.prototype.markAllRead = function () {
|
|
var model = this,
|
|
data = {
|
|
action: 'echomarkread',
|
|
uselang: this.userLang,
|
|
sections: $.isArray( this.type ) ? this.type.join( '|' ) : this.type
|
|
};
|
|
|
|
return this.api.postWithToken( 'edit', data )
|
|
.then( function ( result ) {
|
|
return OO.getProp( result.query, 'echomarkread', model.type, 'rawcount' ) || 0;
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler.prototype.markItemRead = function ( itemId ) {
|
|
var model = this,
|
|
data = {
|
|
action: 'echomarkread',
|
|
uselang: this.userLang,
|
|
list: itemId
|
|
};
|
|
|
|
return this.api.postWithToken( 'edit', data )
|
|
.then( function ( result ) {
|
|
return OO.getProp( result.query, 'echomarkread', model.type, 'rawcount' ) || 0;
|
|
} );
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.dm.LocalAPIHandler.prototype.fetchUnreadCount = function () {
|
|
var apiData = {
|
|
action: 'query',
|
|
meta: 'notifications',
|
|
notsections: $.isArray( this.type ) ? this.type.join( '|' ) : this.type,
|
|
notmessageunreadfirst: 1,
|
|
notlimit: this.limit,
|
|
notprop: 'index|count',
|
|
uselang: this.userLang
|
|
};
|
|
|
|
return this.api.get( apiData )
|
|
.then( function ( result ) {
|
|
return OO.getProp( result.query, 'notifications', 'rawcount' ) || 0;
|
|
} );
|
|
};
|
|
} )( mediaWiki, jQuery );
|