mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-24 07:54:13 +00:00
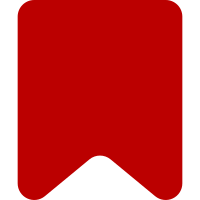
Pass a relevant number of unread notifications to the messages 'echo-mark-all-as-read' and 'echo-mark-wiki-as-read' each time they are used, in order to facilitate the use of PLURAL in the messages. This allows translators to say e.g. "mark both as read" when there are two unread notifications, for instance. Bug: T321462 Change-Id: Ida65be2d7f2663d9802f71c7ea1eb588db8cd254
89 lines
2.8 KiB
JavaScript
89 lines
2.8 KiB
JavaScript
var mobile = mw.mobileFrontend.require( 'mobile.startup' ),
|
|
util = mobile.util,
|
|
View = mobile.View,
|
|
promisedView = mobile.promisedView;
|
|
|
|
/**
|
|
* This callback is displayed as a global member.
|
|
*
|
|
* @callback FunctionCountChangeCallback
|
|
* @param {number} count a capped (0-99 or 99+) count
|
|
*/
|
|
|
|
/**
|
|
* List of notifications
|
|
*
|
|
* @param {mw.echo} echo class
|
|
* @param {OO.ui.ButtonWidget} markAllReadButton - a button that will be associated with the
|
|
* read status of the notifications list.
|
|
* @param {FunctionCountChangeCallback} onCountChange callback.
|
|
* @param {Function} onNotificationListRendered a function that is called when the
|
|
* notifications list has fully rendered (taking no arguments)
|
|
* @return {View}
|
|
*/
|
|
function notificationsList( echo, markAllReadButton, onCountChange, onNotificationListRendered ) {
|
|
var wrapperWidget,
|
|
maxNotificationCount = require( './config.json' ).EchoMaxNotificationCount,
|
|
echoApi = new echo.api.EchoApi(),
|
|
unreadCounter = new echo.dm.UnreadNotificationCounter( echoApi, 'all', maxNotificationCount ),
|
|
modelManager = new echo.dm.ModelManager( unreadCounter, { type: [ 'message', 'alert' ] } ),
|
|
controller = new echo.Controller(
|
|
echoApi,
|
|
modelManager,
|
|
{
|
|
type: [ 'message', 'alert' ]
|
|
}
|
|
),
|
|
markAsReadHandler = function () {
|
|
markAllReadButton.toggle(
|
|
controller.manager.hasLocalUnread()
|
|
);
|
|
markAllReadButton.setTitle(
|
|
mw.msg( 'echo-mark-all-as-read', unreadCounter.getCount() )
|
|
);
|
|
},
|
|
// Create a container which will be revealed when "more options" (...)
|
|
// is clicked on a notification. Hidden by default.
|
|
$moreOptions = util.parseHTML( '<div>' )
|
|
.addClass( 'notifications-overlay-overlay position-fixed' );
|
|
|
|
echo.config.maxPrioritizedActions = 1;
|
|
|
|
wrapperWidget = new echo.ui.NotificationsWrapper( controller, modelManager, {
|
|
$overlay: $moreOptions
|
|
} );
|
|
|
|
// Events
|
|
unreadCounter.on( 'countChange', function ( count ) {
|
|
onCountChange(
|
|
controller.manager.getUnreadCounter().getCappedNotificationCount( count )
|
|
);
|
|
markAsReadHandler();
|
|
} );
|
|
markAllReadButton.on( 'click', function () {
|
|
var numNotifications = controller.manager.getLocalUnread().length;
|
|
|
|
controller.markLocalNotificationsRead()
|
|
.then( function () {
|
|
mw.notify( mw.msg( 'echo-mark-all-as-read-confirmation', numNotifications ) );
|
|
markAllReadButton.toggle( false );
|
|
}, function () {
|
|
markAllReadButton.toggle( false );
|
|
} );
|
|
} );
|
|
|
|
return promisedView(
|
|
// Populate notifications
|
|
wrapperWidget.populate().then( function () {
|
|
controller.updateSeenTime();
|
|
onNotificationListRendered();
|
|
markAsReadHandler();
|
|
// Connect event here as we know that everything loaded correctly
|
|
modelManager.on( 'update', markAsReadHandler );
|
|
return View.make( {}, [ wrapperWidget.$element, $moreOptions ] );
|
|
} )
|
|
);
|
|
}
|
|
|
|
module.exports = notificationsList;
|