mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-15 11:59:11 +00:00
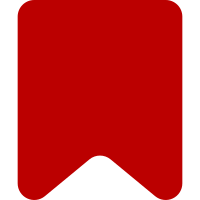
* Declare variables inline, as per the current code conventions. * Convert ad-hoc cases objects into native QUnit.test.each(). This makes for shorter and cleaner code, as well as for more detailed test reporting, and removes the need to manually construct assertion messages based on test case prefix string etc. * Start adopting ES5 Array.forEach in a few places where otherwise ESLint would complain about variable name clashes. * Future proof the test module names, by stripping the global variable namespace that some classes still use, matching packageFiles convention as used for NotificationBadge.js and its tests already, by specifying only the bundle name and the exported class name. Note that the QUnit UI does fuzzy matching so filtering works the same either way, e.g. "echodmfilter" will match "ext.echo.dm - FilterModel". Change-Id: I49858dd2c95d0869f2cd15693f05c38312a9f710
55 lines
1.2 KiB
JavaScript
55 lines
1.2 KiB
JavaScript
QUnit.module( 'ext.echo.dm - SeenTimeModel' );
|
|
|
|
QUnit.test( '.getTypes()', function ( assert ) {
|
|
var model = new mw.echo.dm.SeenTimeModel();
|
|
|
|
assert.deepEqual(
|
|
model.getTypes(),
|
|
[ 'alert', 'message' ],
|
|
'Default model has both types'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( '.setSeenTime() reflected', function ( assert ) {
|
|
var model;
|
|
|
|
model = new mw.echo.dm.SeenTimeModel();
|
|
model.setSeenTime( '20160101010000' );
|
|
|
|
assert.deepEqual(
|
|
model.getSeenTime(),
|
|
'20160101010000',
|
|
'Model sets seen time for both types'
|
|
);
|
|
|
|
model = new mw.echo.dm.SeenTimeModel( { types: 'alert' } );
|
|
model.setSeenTime( '20160101010001' );
|
|
|
|
assert.deepEqual(
|
|
model.getSeenTime(),
|
|
'20160101010001',
|
|
'Alerts seen time model returns correct time'
|
|
);
|
|
} );
|
|
|
|
QUnit.test( '.setSeenTime() events', function ( assert ) {
|
|
var results = [];
|
|
var model = new mw.echo.dm.SeenTimeModel();
|
|
|
|
// Attach a listener
|
|
model.on( 'update', function ( time ) {
|
|
results.push( time );
|
|
} );
|
|
|
|
// Trigger events
|
|
model.setSeenTime( '1' ); // [ '1' ]
|
|
model.setSeenTime( '2' ); // [ '1', '2' ]
|
|
model.setSeenTime( '2' ); // (no change, no event) [ '1', '2' ]
|
|
|
|
assert.deepEqual(
|
|
results,
|
|
[ '1', '2' ],
|
|
'events emitted'
|
|
);
|
|
} );
|