mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-30 18:45:07 +00:00
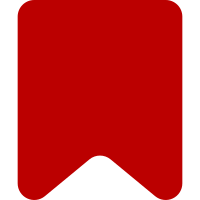
Provides a basic push notifier implementation. Since the push service is not yet in place, all it does for now is log debug output when a notification is to be sent. To register the push notifier, add the following configuration to LocalSettings.php: $wgEchoNotifiers['push'] = [ 'EchoPush\\PushNotifier', 'notifyWithPush' ]; $wgDefaultNotifyTypeAvailability['push'] = true; $wgNotifyTypeAvailabilityByCategory['system']['push'] = false; $wgNotifyTypeAvailabilityByCategory['system-noemail']['push'] = false; We'll register the notifier in configuration for now, rather than hard-coding the default in extension.json, in order to have control over when and where it rolls out (beta vs. prod, as well as which wikis). Since the push notifier implementation depends on jobs being processed by the job queue, I also recommend adding the following configuration setting to ensure that all pending jobs are processed at the end of each web request: // ensure all pending jobs are processed when a web request completes $wgJobRunRate = PHP_INT_MAX; Bug: T252899 Change-Id: Ie7f222443045d30620ff297b006104ef18a074a8
41 lines
1 KiB
PHP
41 lines
1 KiB
PHP
<?php
|
|
|
|
use EchoPush\NotificationServiceClient;
|
|
use EchoPush\SubscriptionManager;
|
|
use MediaWiki\MediaWikiServices;
|
|
|
|
class EchoServices {
|
|
|
|
/** @var MediaWikiServices */
|
|
private $services;
|
|
|
|
/** @return EchoServices */
|
|
public static function getInstance(): EchoServices {
|
|
return new self( MediaWikiServices::getInstance() );
|
|
}
|
|
|
|
/**
|
|
* @param MediaWikiServices $services
|
|
* @return EchoServices
|
|
*/
|
|
public static function wrap( MediaWikiServices $services ): EchoServices {
|
|
return new self( $services );
|
|
}
|
|
|
|
/** @param MediaWikiServices $services */
|
|
public function __construct( MediaWikiServices $services ) {
|
|
$this->services = $services;
|
|
}
|
|
|
|
/** @return NotificationServiceClient */
|
|
public function getPushNotificationServiceClient(): NotificationServiceClient {
|
|
return $this->services->getService( 'EchoPushNotificationServiceClient' );
|
|
}
|
|
|
|
/** @return SubscriptionManager */
|
|
public function getPushSubscriptionManager(): SubscriptionManager {
|
|
return $this->services->getService( 'EchoPushSubscriptionManager' );
|
|
}
|
|
|
|
}
|