mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-13 17:57:21 +00:00
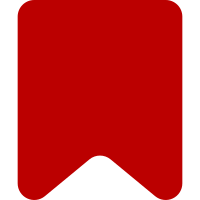
isRegistered is part of the slick UserIdentity interface, i.e. it's the more "canonical" form. This change makes it a bit easier to move away from using the huge (4000+ LOC) User class everywhere, in favor of the UserIdentity interface, where possible. This patch is meant as a small step towards this goal. I tried to replace some usages of User type hints already, but prefer to go in small, incremental steps. Change-Id: I039c7a18672dfb6ea9507752bce9ea754babd690
67 lines
1.8 KiB
PHP
67 lines
1.8 KiB
PHP
<?php
|
|
|
|
// This is a GET module, not a POST module, for multi-DC support. See T222851.
|
|
// Note that this module doesn't write to the database, only to the seentime cache.
|
|
class ApiEchoMarkSeen extends ApiBase {
|
|
|
|
public function execute() {
|
|
// To avoid API warning, register the parameter used to bust browser cache
|
|
$this->getMain()->getVal( '_' );
|
|
|
|
$user = $this->getUser();
|
|
if ( !$user->isRegistered() ) {
|
|
$this->dieWithError( 'apierror-mustbeloggedin-generic', 'login-required' );
|
|
}
|
|
|
|
$params = $this->extractRequestParams();
|
|
$timestamp = wfTimestamp( TS_MW );
|
|
$seenTime = EchoSeenTime::newFromUser( $user );
|
|
$seenTime->setTime( $timestamp, $params['type'] );
|
|
|
|
if ( $params['timestampFormat'] === 'ISO_8601' ) {
|
|
$outputTimestamp = wfTimestamp( TS_ISO_8601, $timestamp );
|
|
} else {
|
|
// MW
|
|
$this->addDeprecation(
|
|
'apiwarn-echo-deprecation-timestampformat',
|
|
'action=echomarkseen×tampFormat=MW'
|
|
);
|
|
|
|
$outputTimestamp = $timestamp;
|
|
}
|
|
|
|
$this->getResult()->addValue( 'query', $this->getModuleName(), [
|
|
'result' => 'success',
|
|
'timestamp' => $outputTimestamp,
|
|
] );
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return [
|
|
'type' => [
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
ApiBase::PARAM_TYPE => [ 'alert', 'message', 'all' ],
|
|
],
|
|
'timestampFormat' => [
|
|
// Not using the TS constants, since clients can't.
|
|
ApiBase::PARAM_DFLT => 'MW',
|
|
ApiBase::PARAM_TYPE => [ 'ISO_8601', 'MW' ],
|
|
],
|
|
];
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
* @return string[]
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return [
|
|
'action=echomarkseen&type=all' => 'apihelp-echomarkseen-example-1',
|
|
];
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Special:MyLanguage/Echo_(Notifications)/API';
|
|
}
|
|
}
|