mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
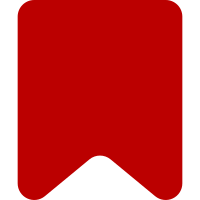
Formatters based on presentation models for individual event emails and digest (daily, weekly) plain text emails. Bug: T121067 Change-Id: I4eceaf521315adab7429a8a73ffca70ebcddab86
79 lines
2.3 KiB
PHP
79 lines
2.3 KiB
PHP
<?php
|
|
|
|
class EchoPlainTextDigestEmailFormatter extends EchoEventDigestFormatter {
|
|
|
|
/**
|
|
* @var string 'daily' or 'weekly'
|
|
*/
|
|
protected $digestMode;
|
|
|
|
public function __construct( User $user, Language $language, $digestMode ) {
|
|
parent::__construct( $user, $language );
|
|
$this->digestMode = $digestMode;
|
|
}
|
|
|
|
/**
|
|
* @param EchoEventPresentationModel[] $models
|
|
* @return array of the following format:
|
|
* [ 'body' => formatted email body,
|
|
* 'subject' => formatted email subject ]
|
|
*/
|
|
protected function formatModels( array $models ) {
|
|
$content = array();
|
|
foreach ( $models as $model ) {
|
|
$content[$model->getCategory()][] = EchoDiscussionParser::htmlToText( $model->getHeaderMessage()->parse() );
|
|
}
|
|
|
|
ksort( $content );
|
|
|
|
// echo-email-batch-body-intro-daily
|
|
// echo-email-batch-body-intro-weekly
|
|
$text = $this->msg( 'echo-email-batch-body-intro-' . $this->digestMode )
|
|
->params( $this->user->getName() )->text();
|
|
|
|
// Does this need to be a message?
|
|
$bullet = $this->msg( 'echo-email-batch-bullet' )->text();
|
|
|
|
foreach ( $content as $type => $items ) {
|
|
$text .= "\n\n--\n\n";
|
|
$text .= $this->getCategoryTitle( $type, count( $items ) );
|
|
$text .= "\n";
|
|
foreach ( $items as $item ) {
|
|
$text .= "\n$bullet $item";
|
|
}
|
|
}
|
|
|
|
$colon = $this->msg( 'colon-separator' )->text();
|
|
$text .= "\n\n--\n\n";
|
|
$viewAll = $this->msg( 'echo-email-batch-link-text-view-all-notifications' )->text();
|
|
$link = SpecialPage::getTitleFor( 'Notifications' )->getFullURL( '', false, PROTO_CANONICAL );
|
|
$text .= "$viewAll$colon <$link>";
|
|
|
|
$plainTextFormatter = new EchoPlainTextEmailFormatter( $this->user, $this->language );
|
|
|
|
$text .= "\n\n{$plainTextFormatter->getFooter()}";
|
|
|
|
// echo-email-batch-subject-daily
|
|
// echo-email-batch-subject-weekly
|
|
$subject = $this->msg( 'echo-email-batch-subject-' . $this->digestMode )
|
|
->numParams( count( $models ), count( $models ) )
|
|
->text();
|
|
|
|
return array(
|
|
'subject' => $subject,
|
|
'body' => $text,
|
|
);
|
|
}
|
|
|
|
/**
|
|
* @param string $type Notification type
|
|
* @param int $count Number of notifications in this type's section
|
|
* @return string Formatted category section title
|
|
*/
|
|
private function getCategoryTitle( $type, $count ) {
|
|
return $this->msg( "echo-category-title-$type" )
|
|
->numParams( $count )
|
|
->text();
|
|
}
|
|
}
|