mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
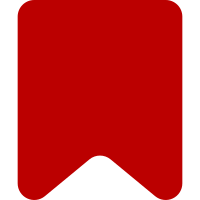
Previously, getNotificationCount() only looked at local notifications, and foreign notifications were added in separately by getMessageCount() and getAlertCount(). This didn't make any sense and resulted in counter-intuitive things like I4d49b543. Instead, add a $global flag to getNotificationCount(). If $global=false, the local count is returned as before, but if $global=true, the global count (=local+foreign) is returned. If $global is omitted, the user's cross-wiki notification preference determines which is returned. Update getLastUnreadNotificationCount() in the same way, since it had the same issues. Also add caching for global counts and timestamps, using a global memc key. Bug: T133623 Change-Id: If78bfc710acd91a075771b565cc99f4c302a104d
112 lines
2.9 KiB
PHP
112 lines
2.9 KiB
PHP
<?php
|
|
|
|
class ApiEchoMarkRead extends ApiBase {
|
|
|
|
public function execute() {
|
|
// To avoid API warning, register the parameter used to bust browser cache
|
|
$this->getMain()->getVal( '_' );
|
|
|
|
$user = $this->getUser();
|
|
if ( $user->isAnon() ) {
|
|
$this->dieUsage( 'Login is required', 'login-required' );
|
|
}
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( $user );
|
|
|
|
$params = $this->extractRequestParams();
|
|
|
|
// There is no need to trigger markRead if all notifications are read
|
|
if ( $notifUser->getLocalNotificationCount() > 0 ) {
|
|
if ( count( $params['list'] ) ) {
|
|
// Make sure there is a limit to the update
|
|
$notifUser->markRead( array_slice( $params['list'], 0, ApiBase::LIMIT_SML2 ) );
|
|
// Mark all as read
|
|
} elseif ( $params['all'] ) {
|
|
$notifUser->markAllRead();
|
|
// Mark all as read for sections
|
|
} elseif ( $params['sections'] ) {
|
|
$notifUser->markAllRead( $params['sections'] );
|
|
}
|
|
}
|
|
|
|
// Mark as unread
|
|
if ( count( $params['unreadlist'] ) > 0 ) {
|
|
// Make sure there is a limit to the update
|
|
$notifUser->markUnRead( array_slice( $params['unreadlist'], 0, ApiBase::LIMIT_SML2 ) );
|
|
}
|
|
|
|
$result = array(
|
|
'result' => 'success'
|
|
);
|
|
$rawCount = 0;
|
|
foreach ( EchoAttributeManager::$sections as $section ) {
|
|
$rawSectionCount = $notifUser->getNotificationCount( /* $tryCache = */true, DB_SLAVE, $section );
|
|
$result[$section]['rawcount'] = $rawSectionCount;
|
|
$result[$section]['count'] = EchoNotificationController::formatNotificationCount( $rawSectionCount );
|
|
$rawCount += $rawSectionCount;
|
|
}
|
|
|
|
$result += array(
|
|
'rawcount' => $rawCount,
|
|
'count' => EchoNotificationController::formatNotificationCount( $rawCount ),
|
|
);
|
|
$this->getResult()->addValue( 'query', $this->getModuleName(), $result );
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return array(
|
|
'list' => array(
|
|
ApiBase::PARAM_ISMULTI => true,
|
|
),
|
|
'unreadlist' => array(
|
|
ApiBase::PARAM_ISMULTI => true,
|
|
),
|
|
'all' => array(
|
|
ApiBase::PARAM_REQUIRED => false,
|
|
ApiBase::PARAM_TYPE => 'boolean'
|
|
),
|
|
'sections' => array(
|
|
ApiBase::PARAM_TYPE => EchoAttributeManager::$sections,
|
|
ApiBase::PARAM_ISMULTI => true,
|
|
),
|
|
'token' => array(
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
),
|
|
);
|
|
}
|
|
|
|
public function needsToken() {
|
|
return 'csrf';
|
|
}
|
|
|
|
public function getTokenSalt() {
|
|
return '';
|
|
}
|
|
|
|
public function mustBePosted() {
|
|
return true;
|
|
}
|
|
|
|
public function isWriteMode() {
|
|
return true;
|
|
}
|
|
|
|
/**
|
|
* @see ApiBase::getExamplesMessages()
|
|
*/
|
|
protected function getExamplesMessages() {
|
|
return array(
|
|
'action=echomarkread&list=8'
|
|
=> 'apihelp-echomarkread-example-1',
|
|
'action=echomarkread&all=true'
|
|
=> 'apihelp-echomarkread-example-2',
|
|
'action=echomarkread&unreadlist=1'
|
|
=> 'apihelp-echomarkread-example-3',
|
|
);
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Echo_(Notifications)/API';
|
|
}
|
|
}
|