mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-12-04 20:28:49 +00:00
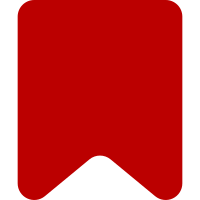
Core change I2793a3f2 changes API handling in a way that needs updates to extensions for proper operation: * needsToken() now returns a string * Most custom token types are being replaced with a 'csrf' token (the former 'edit' token); any others need a new hook. * All tokens must use a static salt. Compat with web UI using non-static tokens is supported and also serves to handle the now-deprecated token fetching. * Documentation in getParamDescription() should return a string (not array) for 'token', as the signal to core that it should be replaced with a standardized message. When compatibility with earlier versions of MediaWiki is no longer maintained, the entry for 'token' from getAllowedParams() and getParamDescription() may be removed, as may getTokenSalt(). This patch leaves them in place. Note this is intended to be compatible with earlier versions of MediaWiki, and so should be safe to merge before the core change. Change-Id: I7cbdc5a7119cdfeadc1fe55469065a44c228a006
108 lines
2.6 KiB
PHP
108 lines
2.6 KiB
PHP
<?php
|
|
|
|
class ApiEchoMarkRead extends ApiBase {
|
|
|
|
public function execute() {
|
|
// To avoid API warning, register the parameter used to bust browser cache
|
|
$this->getMain()->getVal( '_' );
|
|
|
|
$user = $this->getUser();
|
|
if ( $user->isAnon() ) {
|
|
$this->dieUsage( 'Login is required', 'login-required' );
|
|
}
|
|
|
|
$notifUser = MWEchoNotifUser::newFromUser( $user );
|
|
|
|
$params = $this->extractRequestParams();
|
|
|
|
// There is no need to trigger markRead if all notifications are read
|
|
if ( $notifUser->getNotificationCount() > 0 ) {
|
|
if ( count( $params['list'] ) ) {
|
|
// Make sure there is a limit to the update
|
|
$notifUser->markRead( array_slice( $params['list'], 0, ApiBase::LIMIT_SML2 ) );
|
|
} elseif ( $params['all'] ) {
|
|
$notifUser->markAllRead();
|
|
}
|
|
}
|
|
|
|
$rawCount = $notifUser->getNotificationCount();
|
|
|
|
$result = array(
|
|
'result' => 'success'
|
|
);
|
|
$rawCount = 0;
|
|
foreach ( EchoAttributeManager::$sections as $section ) {
|
|
$rawSectionCount = $notifUser->getNotificationCount( /* $tryCache = */true, DB_SLAVE, $section );
|
|
$result[$section]['rawcount'] = $rawSectionCount;
|
|
$result[$section]['count'] = EchoNotificationController::formatNotificationCount( $rawSectionCount );
|
|
$rawCount += $rawSectionCount;
|
|
}
|
|
|
|
$result += array(
|
|
'rawcount' => $rawCount,
|
|
'count' => EchoNotificationController::formatNotificationCount( $rawCount ),
|
|
);
|
|
$this->getResult()->addValue( 'query', $this->getModuleName(), $result );
|
|
}
|
|
|
|
public function getAllowedParams() {
|
|
return array(
|
|
'list' => array(
|
|
ApiBase::PARAM_ISMULTI => true,
|
|
),
|
|
'all' => array(
|
|
ApiBase::PARAM_REQUIRED => false,
|
|
ApiBase::PARAM_TYPE => 'boolean'
|
|
),
|
|
'token' => array(
|
|
ApiBase::PARAM_REQUIRED => true,
|
|
),
|
|
'uselang' => null
|
|
);
|
|
}
|
|
|
|
public function getParamDescription() {
|
|
return array(
|
|
'list' => 'A list of notification IDs to mark as read',
|
|
'all' => "If set to true, marks all of a user's notifications as read",
|
|
'token' => 'edit token',
|
|
'uselang' => 'the desired language to format the output'
|
|
);
|
|
}
|
|
|
|
public function needsToken() {
|
|
return 'csrf';
|
|
}
|
|
|
|
public function getTokenSalt() {
|
|
return '';
|
|
}
|
|
|
|
public function mustBePosted() {
|
|
return true;
|
|
}
|
|
|
|
public function isWriteMode() {
|
|
return true;
|
|
}
|
|
|
|
public function getDescription() {
|
|
return 'Mark notifications as read for the current user';
|
|
}
|
|
|
|
public function getExamples() {
|
|
return array(
|
|
'api.php?action=echomarkread&list=8',
|
|
'api.php?action=echomarkread&all=true'
|
|
);
|
|
}
|
|
|
|
public function getHelpUrls() {
|
|
return 'https://www.mediawiki.org/wiki/Echo_(notifications)/API';
|
|
}
|
|
|
|
public function getVersion() {
|
|
return __CLASS__ . '-0.1';
|
|
}
|
|
}
|