mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-24 10:49:37 +00:00
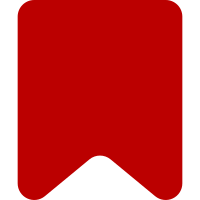
The workflow to format a notification is * Get EchoEvent, User, and Language * Get EchoEventFormatter implementation for notification type ** EchoEventFormatter returns structured data about each part of the notification (header, body, primary link, secondary link(s)) * Each output type will have a formatter class (e.g. EchoSpecialNotificationsFormatter, EchoPlainTextEmailFormatter) which takes a EchoEventPresentationModel and generates whatever it wants (HTML, plain-text email, etc). Included is an example conversion of the user-rights and mention formatters. The previous infrastructure will remain in place for backwards compatability until other extensions can be updated. Bug: T107823 Change-Id: I4397872a7ec062148dfcb066ddd8ab83f40486ac
74 lines
1.9 KiB
PHP
74 lines
1.9 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Formatter for 'user-rights' notifications
|
|
*/
|
|
class EchoUserRightsFormatter extends EchoBasicFormatter {
|
|
|
|
/**
|
|
* @param $event EchoEvent
|
|
* @param $param string
|
|
* @param $message Message
|
|
* @param $user User
|
|
*/
|
|
protected function processParam( $event, $param, $message, $user ) {
|
|
$extra = $event->getExtra();
|
|
switch ( $param ) {
|
|
// List of user rights that are granted or revoked
|
|
case 'user-rights-list':
|
|
$lang = $this->getLanguage();
|
|
|
|
$list = array();
|
|
|
|
foreach ( array( 'add', 'remove' ) as $action ) {
|
|
if ( isset( $extra[$action] ) && $extra[$action] ) {
|
|
|
|
// Get the localized group names, bug 55338
|
|
$groups = array();
|
|
foreach ( $extra[$action] as $group ) {
|
|
$msg = $this->getMessage( 'group-' . $group );
|
|
$groups[] = $msg->isBlank() ? $group : $msg->escaped();
|
|
}
|
|
|
|
// Messages that can be used here:
|
|
// * notification-user-rights-add
|
|
// * notification-user-rights-remove
|
|
$list[] = $this->getMessage( 'notification-user-rights-' . $action )
|
|
->params( $lang->commaList( $groups ), count( $groups ) )
|
|
->escaped();
|
|
}
|
|
}
|
|
$message->params( $lang->semicolonList( $list ) );
|
|
break;
|
|
|
|
default:
|
|
parent::processParam( $event, $param, $message, $user );
|
|
break;
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Helper function for getLink()
|
|
*
|
|
* @param EchoEvent $event
|
|
* @param User $user The user receiving the notification
|
|
* @param String $destination The destination type for the link
|
|
* @return Array including target and query parameters
|
|
*/
|
|
protected function getLinkParams( $event, $user, $destination ) {
|
|
$target = null;
|
|
$query = array();
|
|
// Set up link parameters based on the destination (or pass to parent)
|
|
switch ( $destination ) {
|
|
case 'user-rights-list':
|
|
$target = SpecialPage::getTitleFor( 'Listgrouprights' );
|
|
break;
|
|
default:
|
|
return parent::getLinkParams( $event, $user, $destination );
|
|
}
|
|
|
|
return array( $target, $query );
|
|
}
|
|
}
|
|
|