mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-09-25 03:09:37 +00:00
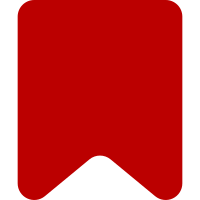
This should have been done from the beginning; the model manager pulls models by their symbolic names. So far, we've used the source for that, but that assumes that two modules always have different sources, and that is absolutely not necessarily the case. For example, internal local bundles will each be a model, but have the same ('local') source. They should still be differentiated in the manager by their names, but the source should state clearly that it is local. For this, the models now have "getName" method and the name is created separately from their source. Items also preserve a reference to their parent's symbolic name so they can provide that for items that require the controller to manipulate a specific model. Change-Id: I8c39d5d28383d11fb330addce21e07d5c424da6f
95 lines
2.9 KiB
JavaScript
95 lines
2.9 KiB
JavaScript
( function ( mw ) {
|
|
/**
|
|
* Single notification item widget for echo popup.
|
|
*
|
|
* @class
|
|
* @extends mw.echo.ui.NotificationItemWidget
|
|
* @mixins OO.ui.mixin.PendingElement
|
|
*
|
|
* @constructor
|
|
* @param {mw.echo.Controller} controller Echo notifications controller
|
|
* @param {mw.echo.dm.NotificationItem} model Notification item model
|
|
* @param {Object} [config] Configuration object
|
|
* @cfg {boolean} [markReadWhenSeen=false] This option is marked as read when it is viewed
|
|
* @cfg {jQuery} [$overlay] A jQuery element functioning as an overlay
|
|
* for popups.
|
|
* @cfg {boolean} [bundle=false] This notification is part of a bundle
|
|
*/
|
|
mw.echo.ui.SingleNotificationItemWidget = function MwEchoUiSingleNotificationItemWidget( controller, model, config ) {
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
mw.echo.ui.SingleNotificationItemWidget.parent.call( this, controller, model, config );
|
|
// Mixin constructors
|
|
OO.ui.mixin.PendingElement.call( this, config );
|
|
|
|
this.controller = controller;
|
|
this.model = model;
|
|
|
|
this.bundle = !!config.bundle;
|
|
this.markReadWhenSeen = !!config.markReadWhenSeen;
|
|
this.$overlay = config.$overlay || this.$element;
|
|
|
|
this.markReadWhenSeen = !!config.markReadWhenSeen;
|
|
|
|
// Toggle 'mark as read' functionality
|
|
this.toggleMarkAsReadButtons( !this.markReadWhenSeen && !this.model.isRead() );
|
|
|
|
// Events
|
|
this.model.connect( this, { update: 'updateDataFromModel' } );
|
|
|
|
// Update read and seen states from the model
|
|
this.updateDataFromModel();
|
|
};
|
|
|
|
/* Initialization */
|
|
|
|
OO.inheritClass( mw.echo.ui.SingleNotificationItemWidget, mw.echo.ui.NotificationItemWidget );
|
|
OO.mixinClass( mw.echo.ui.SingleNotificationItemWidget, OO.ui.mixin.PendingElement );
|
|
|
|
/* Methods */
|
|
mw.echo.ui.SingleNotificationItemWidget.prototype.onPrimaryLinkClick = function () {
|
|
// Log notification click
|
|
|
|
mw.echo.logger.logInteraction(
|
|
mw.echo.Logger.static.actions.notificationClick,
|
|
mw.echo.Logger.static.context.popup,
|
|
this.getModel().getId(),
|
|
this.getModel().getCategory(),
|
|
false,
|
|
// Source of this notification if it is cross-wiki
|
|
// TODO: For notifications in local bundles, we need
|
|
// to consider changing this
|
|
this.bundle ? this.getModel().getSource() : ''
|
|
);
|
|
};
|
|
|
|
/**
|
|
* @inheritdoc
|
|
*/
|
|
mw.echo.ui.SingleNotificationItemWidget.prototype.markRead = function ( isRead ) {
|
|
isRead = isRead !== undefined ? isRead : true;
|
|
|
|
this.controller.markSingleItemRead(
|
|
this.model.getId(),
|
|
this.model.getModelName(),
|
|
this.model.isForeign(),
|
|
!!isRead
|
|
);
|
|
};
|
|
|
|
/**
|
|
* Update item state when the item model changes.
|
|
*
|
|
* @fires sortChange
|
|
*/
|
|
mw.echo.ui.SingleNotificationItemWidget.prototype.updateDataFromModel = function () {
|
|
this.toggleRead( this.model.isRead() );
|
|
this.toggleSeen( this.model.isSeen() );
|
|
|
|
// Emit 'sortChange' so the SortedList can update this
|
|
// item's place in the list
|
|
this.emit( 'sortChange' );
|
|
};
|
|
} )( mediaWiki );
|