mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-12 09:26:05 +00:00
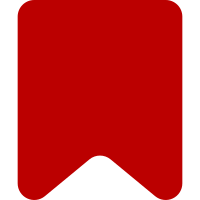
The fade in/out animation is asynchronous. This means that if we are sorting multiple items one after the other, by the time the item faded out, it will be reinserted back into the wrong position, breaking the sorting. This also broke the promise of OO.SortedEmitterList whereby all its items are always in order. The way to fix this was to force a better synchronization with the item order while we hide and show the item in its new place. To do that, a new widget is created as a fake clone of the old one, in the original position of the old one. The original item is then reinserted (while hidden) to the proper location -- preserving order. The fake clone is then faded out, and the real item is then faded in. For this to work properly, the cloned item had to preserve some of the original item's information, like timestamp, foreigness and id. However, since both the real item and the fake new clone have the same details, the clone fakes its ID by adding a fraction to it - promising that the fallback in case of equal timestamps (which happens on the real and cloned items) will still resolve with some decision about the placement of the items rather than (falsely but understandably) decide they are both the same. Since this whole animation is somewhat of a hack, the list now has a configuration parameter to turn the animation on. The animation is on in the popups, but off in the special page. Bug: T141419 Change-Id: Ic7c35e5ddefc51bf7fde497eab36414b4dddcd9e
173 lines
4.5 KiB
JavaScript
173 lines
4.5 KiB
JavaScript
( function ( $, mw ) {
|
|
/**
|
|
* A notifications list organized and separated by dates
|
|
*
|
|
* @class
|
|
* @extends OO.ui.Widget
|
|
* @mixins OO.ui.mixin.PendingElement
|
|
*
|
|
* @constructor
|
|
* @param {mw.echo.Controller} controller Echo controller
|
|
* @param {mw.echo.dm.ModelManager} modelManager Model manager
|
|
* @param {Object} [config] Configuration object
|
|
* @cfg {boolean} [animateSorting=false] Animate the sorting of items
|
|
* @cfg {jQuery} [$overlay] An overlay for the popup menus
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget = function MwEchoUiDatedNotificationsListWidget( controller, modelManager, config ) {
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
mw.echo.ui.DatedNotificationsWidget.parent.call( this, config );
|
|
// Mixin constructors
|
|
OO.ui.mixin.PendingElement.call( this, config );
|
|
|
|
this.manager = modelManager;
|
|
this.controller = controller;
|
|
this.models = {};
|
|
|
|
this.$overlay = config.$overlay || this.$element;
|
|
this.animateSorting = !!config.animateSorting;
|
|
|
|
this.listWidget = new mw.echo.ui.SortedListWidget(
|
|
// Sorting callback
|
|
function ( a, b ) {
|
|
// Reverse sorting
|
|
if ( b.getTimestamp() < a.getTimestamp() ) {
|
|
return -1;
|
|
} else if ( b.getTimestamp() > a.getTimestamp() ) {
|
|
return 1;
|
|
}
|
|
},
|
|
// Config
|
|
{
|
|
classes: [ 'mw-echo-ui-datedNotificationsWidget-group' ],
|
|
$overlay: this.$overlay,
|
|
animated: false
|
|
}
|
|
);
|
|
|
|
// Events
|
|
this.manager.connect( this, {
|
|
update: 'populateFromModel',
|
|
discard: 'onManagerDiscardModel'
|
|
} );
|
|
|
|
this.$element
|
|
.addClass( 'mw-echo-ui-datedNotificationsWidget' )
|
|
.append( this.listWidget.$element );
|
|
|
|
// Initialization
|
|
this.populateFromModel();
|
|
};
|
|
/* Initialization */
|
|
|
|
OO.inheritClass( mw.echo.ui.DatedNotificationsWidget, OO.ui.Widget );
|
|
OO.mixinClass( mw.echo.ui.DatedNotificationsWidget, OO.ui.mixin.PendingElement );
|
|
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.onManagerDiscardModel = function ( modelId ) {
|
|
var group,
|
|
model = this.models[ modelId ],
|
|
list = this.getList();
|
|
|
|
if ( model ) {
|
|
group = list.getItemFromId( model.getName() );
|
|
list.removeItems( [ group ] );
|
|
}
|
|
};
|
|
/**
|
|
* Respond to model removing source group
|
|
*
|
|
* @param {string} source Symbolic name of the source group
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.onModelRemoveSource = function ( source ) {
|
|
var list = this.getList(),
|
|
group = list.getItemFromId( source );
|
|
|
|
list.removeItems( [ group ] );
|
|
};
|
|
|
|
/**
|
|
* Respond to model manager update event.
|
|
* This event means we are repopulating the entire list and the
|
|
* associated models within it.
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.populateFromModel = function ( models ) {
|
|
var modelId, model, subgroupWidget,
|
|
groupWidgets = [];
|
|
|
|
// Detach all attached models
|
|
for ( modelId in this.models ) {
|
|
this.detachModel( modelId );
|
|
}
|
|
|
|
for ( model in models ) {
|
|
// Create SubGroup widgets
|
|
subgroupWidget = new mw.echo.ui.DatedSubGroupListWidget(
|
|
this.controller,
|
|
models[ model ],
|
|
{
|
|
showTitle: true,
|
|
showMarkAllRead: true,
|
|
$overlay: this.$overlay,
|
|
animated: this.animateSorting
|
|
}
|
|
);
|
|
this.attachModel( model, models[ model ] );
|
|
|
|
subgroupWidget.resetItemsFromModel();
|
|
groupWidgets.push( subgroupWidget );
|
|
}
|
|
|
|
// Reset the list and re-add the items
|
|
this.getList().clearItems();
|
|
this.getList().addItems( groupWidgets );
|
|
};
|
|
|
|
/**
|
|
* Attach a model to the widget
|
|
*
|
|
* @param {string} modelId Symbolic name for the model
|
|
* @param {mw.echo.dm.SortedList} model Notifications list model
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.attachModel = function ( modelId, model ) {
|
|
this.models[ modelId ] = model;
|
|
};
|
|
|
|
/**
|
|
* Detach a model from the widget
|
|
*
|
|
* @param {string} modelId Notifications list model
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.detachModel = function ( modelId ) {
|
|
this.models[ modelId ].disconnect( this );
|
|
delete this.models[ modelId ];
|
|
};
|
|
|
|
/**
|
|
* Get the list widget contained in this item
|
|
*
|
|
* @return {mw.echo.ui.SortedListWidget} List widget
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.getList = function () {
|
|
return this.listWidget;
|
|
};
|
|
|
|
/**
|
|
* Get the number of all notifications in all sections of the widget
|
|
*
|
|
* @return {number} The number of all notifications
|
|
*/
|
|
mw.echo.ui.DatedNotificationsWidget.prototype.getAllNotificationCount = function () {
|
|
var i,
|
|
count = 0,
|
|
groups = this.getList().getItems();
|
|
|
|
for ( i = 0; i < groups.length; i++ ) {
|
|
count += groups[ i ].getListWidget().getItemCount();
|
|
}
|
|
|
|
return count;
|
|
};
|
|
|
|
} )( jQuery, mediaWiki );
|