mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-23 23:44:53 +00:00
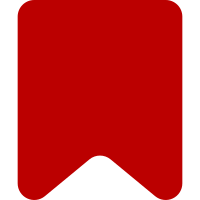
get_debug_type() does the same thing but better (spelling type names in the same way as in type declarations, and including names of object classes and resource types). It was added in PHP 8, but the symfony/polyfill-php80 package provides it while we still support 7.4. Also remove uses of get_class() where the new method already provides the same information. For reference: https://www.php.net/manual/en/function.get-debug-type.php https://www.php.net/manual/en/function.gettype.php Change-Id: I54c2bf287b185e2526b0a8556166fd62182b2235
81 lines
2 KiB
PHP
81 lines
2 KiB
PHP
<?php
|
|
|
|
namespace MediaWiki\Extension\Notifications;
|
|
|
|
use UnexpectedValueException;
|
|
use WANObjectCache;
|
|
|
|
/**
|
|
* Caches an ContainmentList within WANObjectCache to prevent needing
|
|
* to load the nested list from a potentially slow source (mysql, etc).
|
|
*/
|
|
class CachedList implements ContainmentList {
|
|
private const ONE_WEEK = 4233600;
|
|
|
|
/** @var WANObjectCache */
|
|
protected $cache;
|
|
/** @var string */
|
|
protected $partialCacheKey;
|
|
/** @var ContainmentList */
|
|
protected $nestedList;
|
|
/** @var int */
|
|
protected $timeout;
|
|
/** @var string[]|null */
|
|
private $result;
|
|
|
|
/**
|
|
* @param WANObjectCache $cache Bag to stored cached data in.
|
|
* @param string $partialCacheKey Partial cache key, $nestedList->getCacheKey() will be appended
|
|
* to this to construct the cache key used.
|
|
* @param ContainmentList $nestedList The nested EchoContainmentList to cache the result of.
|
|
* @param int $timeout How long in seconds to cache the nested list, defaults to 1 week.
|
|
*/
|
|
public function __construct(
|
|
WANObjectCache $cache,
|
|
$partialCacheKey,
|
|
ContainmentList $nestedList,
|
|
$timeout = self::ONE_WEEK
|
|
) {
|
|
$this->cache = $cache;
|
|
$this->partialCacheKey = $partialCacheKey;
|
|
$this->nestedList = $nestedList;
|
|
$this->timeout = $timeout;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getValues() {
|
|
if ( $this->result ) {
|
|
return $this->result;
|
|
}
|
|
$this->result = $this->cache->getWithSetCallback(
|
|
$this->getCacheKey(),
|
|
$this->timeout,
|
|
function () {
|
|
$result = $this->nestedList->getValues();
|
|
if ( !is_array( $result ) ) {
|
|
throw new UnexpectedValueException( sprintf(
|
|
"Expected array but received '%s' from '%s::getValues'",
|
|
get_debug_type( $result ),
|
|
get_class( $this->nestedList )
|
|
) );
|
|
}
|
|
return $result;
|
|
}
|
|
);
|
|
return $this->result;
|
|
}
|
|
|
|
/**
|
|
* @inheritDoc
|
|
*/
|
|
public function getCacheKey() {
|
|
return $this->cache->makeGlobalKey(
|
|
'echo-containment-list',
|
|
$this->partialCacheKey,
|
|
$this->nestedList->getCacheKey()
|
|
);
|
|
}
|
|
}
|