mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-24 07:54:13 +00:00
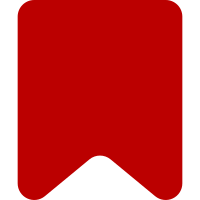
The current initial message in the button label is "Alerts (x)" or "Notices (x)" but when the badge loaded, we changed that to only show the rendered number. This is inconsistent; the badge should always update the label properly, even if it's not visible in most cases. Bug: T173970 Change-Id: I6a5e13fc36ddb1bf467c712fcf49af3b18e582d0
78 lines
2.7 KiB
JavaScript
78 lines
2.7 KiB
JavaScript
( function ( mw, $ ) {
|
|
/**
|
|
* Notification badge button widget for echo popup.
|
|
*
|
|
* @class
|
|
* @extends OO.ui.ButtonWidget
|
|
*
|
|
* @constructor
|
|
* @param {Object} [config] Configuration object
|
|
* @cfg {string} [type] The notification types this button represents;
|
|
* 'message', 'alert' or 'all'
|
|
* @cfg {string} [href] URL the badge links to
|
|
* @cfg {string} [convertedNumber] A converted version of the initial count
|
|
*/
|
|
mw.echo.ui.BadgeLinkWidget = function MwEchoUiBadgeLinkWidget( config ) {
|
|
config = config || {};
|
|
|
|
// Parent constructor
|
|
mw.echo.ui.BadgeLinkWidget.parent.call( this, config );
|
|
|
|
// Mixin constructors
|
|
OO.ui.mixin.LabelElement.call( this, $.extend( { $label: this.$element }, config ) );
|
|
OO.ui.mixin.ButtonElement.call( this, $.extend( { $button: this.$element }, config ) );
|
|
OO.ui.mixin.TitledElement.call( this, $.extend( { $titled: this.$element }, config ) );
|
|
OO.ui.mixin.FlaggedElement.call( this, $.extend( {}, config, { $flagged: this.$element } ) );
|
|
|
|
this.$element
|
|
.addClass( 'mw-echo-notifications-badge' );
|
|
|
|
this.count = 0;
|
|
this.type = config.type || 'alert';
|
|
this.setCount( config.numItems, config.convertedNumber );
|
|
|
|
if ( config.href !== undefined && OO.ui.isSafeUrl( config.href ) ) {
|
|
this.$element.attr( 'href', config.href );
|
|
}
|
|
};
|
|
|
|
OO.inheritClass( mw.echo.ui.BadgeLinkWidget, OO.ui.Widget );
|
|
OO.mixinClass( mw.echo.ui.BadgeLinkWidget, OO.ui.mixin.LabelElement );
|
|
OO.mixinClass( mw.echo.ui.BadgeLinkWidget, OO.ui.mixin.ButtonElement );
|
|
OO.mixinClass( mw.echo.ui.BadgeLinkWidget, OO.ui.mixin.TitledElement );
|
|
OO.mixinClass( mw.echo.ui.BadgeLinkWidget, OO.ui.mixin.FlaggedElement );
|
|
|
|
mw.echo.ui.BadgeLinkWidget.static.tagName = 'a';
|
|
|
|
/**
|
|
* Set the count labels for this button.
|
|
*
|
|
* @param {number} numItems Number of items
|
|
* @param {string} [convertedNumber] Label of the button. Defaults to the default message
|
|
* showing the item number.
|
|
*/
|
|
mw.echo.ui.BadgeLinkWidget.prototype.setCount = function ( numItems, convertedNumber ) {
|
|
convertedNumber = convertedNumber !== undefined ? convertedNumber : numItems;
|
|
|
|
this.$element
|
|
.toggleClass( 'mw-echo-notifications-badge-all-read', !numItems )
|
|
.toggleClass( 'mw-echo-notifications-badge-long-label', convertedNumber.length > 2 )
|
|
.attr( 'data-counter-num', numItems )
|
|
.attr( 'data-counter-text', convertedNumber );
|
|
|
|
this.setLabel( mw.msg(
|
|
this.type === 'alert' ?
|
|
'echo-notification-alert' :
|
|
'echo-notification-notice',
|
|
convertedNumber
|
|
) );
|
|
|
|
if ( this.count !== numItems ) {
|
|
this.count = numItems;
|
|
|
|
// Fire badge count change hook
|
|
mw.hook( 'ext.echo.badge.countChange' ).fire( this.type, this.count, convertedNumber );
|
|
}
|
|
};
|
|
}( mediaWiki, jQuery ) );
|