mirror of
https://gerrit.wikimedia.org/r/mediawiki/extensions/Echo
synced 2024-11-13 17:57:21 +00:00
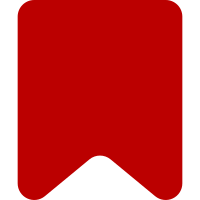
To my knowledge in all the places I'm touching in this patch the new code is functionally identical to the old one. Change-Id: I0ffa96d2f9cb9bf932f68b689244051c96c17ad9
77 lines
1.6 KiB
PHP
77 lines
1.6 KiB
PHP
<?php
|
|
|
|
/**
|
|
* Abstract mapper for model
|
|
*/
|
|
abstract class EchoAbstractMapper {
|
|
|
|
/**
|
|
* Echo database factory
|
|
* @var MWEchoDbFactory
|
|
*/
|
|
protected $dbFactory;
|
|
|
|
/**
|
|
* Event listeners for method like insert/delete
|
|
* @var array[]
|
|
*/
|
|
protected $listeners;
|
|
|
|
/**
|
|
* @param MWEchoDbFactory|null $dbFactory
|
|
*/
|
|
public function __construct( MWEchoDbFactory $dbFactory = null ) {
|
|
if ( $dbFactory === null ) {
|
|
$dbFactory = MWEchoDbFactory::newFromDefault();
|
|
}
|
|
$this->dbFactory = $dbFactory;
|
|
}
|
|
|
|
/**
|
|
* Attach a listener
|
|
*
|
|
* @param string $method Method name
|
|
* @param string $key Identification of the callable
|
|
* @param callable $callable
|
|
* @throws MWException
|
|
*/
|
|
public function attachListener( $method, $key, $callable ) {
|
|
if ( !method_exists( $this, $method ) ) {
|
|
throw new MWException( $method . ' does not exist in ' . get_class( $this ) );
|
|
}
|
|
if ( !isset( $this->listeners[$method] ) ) {
|
|
$this->listeners[$method] = [];
|
|
}
|
|
|
|
$this->listeners[$method][$key] = $callable;
|
|
}
|
|
|
|
/**
|
|
* Detach a listener
|
|
*
|
|
* @param string $method Method name
|
|
* @param string $key identification of the callable
|
|
*/
|
|
public function detachListener( $method, $key ) {
|
|
if ( isset( $this->listeners[$method] ) ) {
|
|
unset( $this->listeners[$method][$key] );
|
|
}
|
|
}
|
|
|
|
/**
|
|
* Get the listener for a method
|
|
*
|
|
* @param string $method
|
|
* @return callable[]
|
|
* @throws MWException
|
|
*/
|
|
public function getMethodListeners( $method ) {
|
|
if ( !method_exists( $this, $method ) ) {
|
|
throw new MWException( $method . ' does not exist in ' . get_class( $this ) );
|
|
}
|
|
|
|
return $this->listeners[$method] ?? [];
|
|
}
|
|
|
|
}
|